How can I create a piecewise inline function in MATLAB?
Solution 1
Unfortunately, MATLAB doesn't have a ternary operator which would make this sort of thing easier, but to expand slightly on gnovice's approach, you could create an anonymous function like so:
fh = @(x) ( 2 .* ( x <= 0.5 ) - 1 )
In general, anonymous functions are more powerful than inline function objects, and allow you to create closures etc.
Solution 2
If you really want to make an inline function (as opposed to an anonymous function), then the following would probably be the simplest way:
f = inline('2.*(x <= 0.5)-1');
However, as pointed out in the other answers, anonymous functions are more commonly used and are more efficient:
f = @(x) (2.*(x <= 0.5)-1);
Solution 3
I just had to solve that problem, and I think the easiest thing to do is use anonymous functions. Say that you have a piecewise function:
when x<0 : x^2 + 3x
when 0<=x<=4: e^x
when x>4 : log(x)
I'd first define logical masks for each piecewise region:
PIECE1 = @(x) x<0
PIECE2 = @(x) x>=0 & x<=4
PIECE3 = @(x) x>4
Then I'd put them all together:
f = @(x) PIECE1(x).*(x.^2+3*x) + PIECE2(x).*exp(x) + PIECE3(x).*log(x)
x = -10:.1:10
figure;
plot(x,f(x))
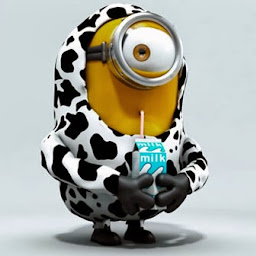
Robert LaFondue
Updated on November 07, 2020Comments
-
Robert LaFondue over 3 years
I have a function in MATLAB which takes another function as an argument. I would like to somehow define a piecewise inline function that can be passed in. Is this somehow possible in MATLAB?
Edit: The function I would like to represent is:
f(x) = { 1.0, 0.0 <= x <= 0.5, -1.0, 0.5 < x <= 1.0 where 0.0 <= x <= 1.0