How can I customize the selection state of my UICollectionViewCell subclass?
Solution 1
Add a public method performSelectionAnimations
to the definition of MyCollectionViewCell
that changes the desired UIImageView
and performs the desired animation. Then call it from collectionView:didSelectItemAtIndexPath:
.
So in MyCollectionViewCell.m:
- (void)performSelectionAnimations {
// Swap the UIImageView
...
// Light bounce animation
...
}
And in your UICollectionViewController
:
- (void)collectionView:(UICollectionView *)collectionView didSelectItemAtIndexPath:(NSIndexPath *)indexPath {
MyCollectionViewCell *cell = (MyCollectionViewCell *)[collectionView cellForItemAtIndexPath:indexPath];
[cell performSelectionAnimations];
}
Notice I've taken out the call to [cell setSelected:YES]
, since that should already be taken care of by the UICollectionView. From the documentation:
The preferred way to select the cell and highlight it is to use the selection methods of the collection view object.
Solution 2
In your custom UICollectionViewCell
subclass, you can implement didSet
on the isSelected
property.
Swift 3:
override var isSelected: Bool {
didSet {
if isSelected {
// animate selection
} else {
// animate deselection
}
}
}
Swift 2:
override var selected: Bool {
didSet {
if self.selected {
// animate selection
} else {
// animate deselection
}
}
}
Solution 3
In your custom UICollectionViewCell subclass you could override the setSelected:
as so:
- (void)setSelected:(BOOL)selected {
[super setSelected:selected];
if (selected) {
[self animateSelection];
} else {
[self animateDeselection];
}
}
I have found that on repeated touches this method is called on a cell even if it's already selected so you may want to just check that you are really changing state before firing unwanted animations.
Solution 4
If you want to show animation on selection then following method might helpful to you :
- (void)collectionView:(UICollectionView *)collectionView didSelectItemAtIndexPath:(NSIndexPath *)indexPath {
NSLog(@"cell #%d was selected", indexPath.row);
// animate the cell user tapped on
UICollectionViewCell *cell = [collectionView cellForItemAtIndexPath:indexPath];
[UIView animateWithDuration:0.8
delay:0
options:(UIViewAnimationOptionAllowUserInteraction)
animations:^{
[cell setBackgroundColor:UIColorFromRGB(0x05668d)];
}
completion:^(BOOL finished){
[cell setBackgroundColor:[UIColor clearColor]];
}
];
}
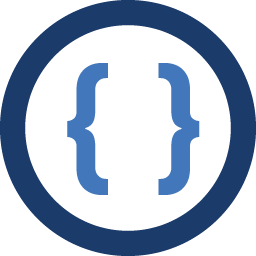
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I have a custom UICollectionViewCell subclass that overwrites
initWithFrame:
andlayoutSubviews
to setup its views. However, I'm now trying to do two things which I'm having trouble with.1) I'm trying to customize the state of the
UICollectionViewCell
upon selection. For example, I want to change one of the images in anUIImageView
in theUICollectionViewCell
.2) I want to animate (light bounce) the
UIImage
in theUICollectionViewCell
.Can anyone point me in the right direction?
- (void)collectionView:(UICollectionView *)collectionView didSelectItemAtIndexPath:(NSIndexPath *)indexPath { MyCollectionViewCell *cell = (MyCollectionViewCell *)[collectionView cellForItemAtIndexPath:indexPath]; [cell setSelected:YES]; }