How can I cut an image from the bottom using PHP?
Solution 1
Here you go.
To change the name of the image, change $in_filename (currently 'source.jpg'). You can use URLs in there as well, although obviously that will perform worse.
Change the $new_height variable to set how much of the bottom you want cropped.
Play around with $offset_x, $offset_y, $new_width and $new_height, and you'll figure it out.
Please let me know that it works. :)
Hope it helps!
<?php
$in_filename = 'source.jpg';
list($width, $height) = getimagesize($in_filename);
$offset_x = 0;
$offset_y = 0;
$new_height = $height - 15;
$new_width = $width;
$image = imagecreatefromjpeg($in_filename);
$new_image = imagecreatetruecolor($new_width, $new_height);
imagecopy($new_image, $image, 0, 0, $offset_x, $offset_y, $width, $height);
header('Content-Type: image/jpeg');
imagejpeg($new_image);
?>
Solution 2
You may use the GD Image Library to manipulate images in PHP. The function you're looking for is imagecopy()
, which copies part of an image onto another. Here's an example from PHP.net that does roughly what you describe:
<?php
$width = 50;
$height = 50;
$source_x = 0;
$source_y = 0;
// Create images
$source = imagecreatefromjpeg('source.jpg');
$new = imagecreatetruecolor($width, $height);
// Copy
imagecopy($source, $new, 0, 0, $source_x, $source_y, $width, $height);
// Output image
header('Content-Type: image/jpeg');
imagejpeg($new);
?>
To crop the source image, change the $source_x
and $source_y
variables to your liking.
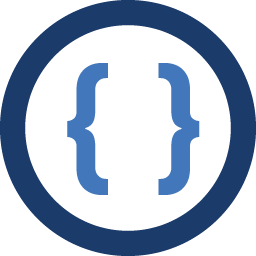
Admin
Updated on June 20, 2022Comments
-
Admin almost 2 years
I want to take out the text in the bottom of an image. How can I cut it from bottom ...say 10 pixels to cut from bottom.
I want do this in PHP. I have lots of images that have text in the bottom.
Is there a way to do it?
-
Admin about 14 yearscan someone please show me a working code for my need - thanks a lot
-
Johannes Gorset about 14 yearsYou might be mistaking SO for a group of developers who will do your work for free.
-
Johannes Gorset about 14 years@nazir: There's a typo on the last line. Change
imagejpeg($dest)
toimagejpeg($new)
. -
Admin about 14 yearsWow! Thank you so much for the help, that worked. Now i need to save that new image in a dir.. how can i do that?
-
Teekin about 14 yearsI don't want to be a pain in the neck, but would you mind marking my answer as the correct one? :) That's what we're all about around here. ;)
-
Admin about 14 yearsSorry guys i cant vote up for you as my reputation is only 11 :(
-
Teej about 14 years@nazir, you should see a check beside Helgi's answer. You should mark that one if you feel that he's got your question answered.