How can I execute JavaScript code from Python?
21,927
The module Naked
does exactly this. pip install Naked
(or install from source if you prefer) and import the library shell functions as follows:
from Naked.toolshed.shell import execute_js, muterun_js
response = muterun_js('file.js')
if response.exitcode == 0:
print(response.stdout)
else:
sys.stderr.write(response.stderr)
For your particular case, with file.js as
var x = 10;
x = 10 - 5;
console.log(x);
function greet() {
console.log("Hello World!");
}
greet()
the output is '5\nHello World!\n'
, which you can parse as desired.
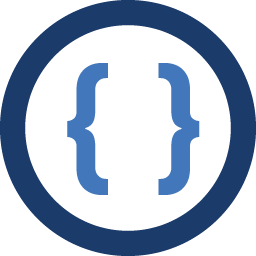
Author by
Admin
Updated on November 19, 2020Comments
-
Admin over 3 years
Lets say that I have this code inside a JavaScript file:
var x = 10; x = 10 - 5; console.log(x); function greet() { console.log("Hello World!"); } greet()
How would I use Python to execute this code and "print"
x
andHello World!
?
Here is some pseudo code that further explains what I'm thinking:# 1. open the script script = open("/path/to/js/files.js", "r") # 2. get the script content script_content = script.read() # 3. close the script file script.close() # 4. execute the script content and "print" "x" and "Hello World!" x = js.exec(script_content)
And, the expected result would look like this:
>>> 5 >>> "Hello World!"
-
Admin over 7 yearsThank you for the answer and help, appreciate it.
-
manan over 7 years@Mango glad I could help :)