How can I get time.Time in Golang protobuf v3 struct?
Solution 1
To obtain a time.Time
from a protobuf field of type google.protobuf.Timestamp
, use the AsTime
method defined on the timestamppb.Timestamp
type.
When producing the insert call into your database, or any other location where a time.Time
is required, call myMsg.UpdateTime.AsTime()
to obtain the required value (where myMsg
is a variable to an instance of the relevant Protobuf message type).
This answer assumes you are using the new Protobuf APIv2 interface, defined in the google.golang.org/protobuf
package, or that you are using the APIv2-compatible implementation of APIv1, defined in package github.com/golang/protobuf
at version v1.20
or greater.
Many projects are still to update to these versions. It is highly recommended that you look to upgrade your code generation and toolchain to benefit from new functionality.
Solution 2
The AsTime method can be used to convert a Timestamp message to a standard Go time.Time value in UTC:
t := ts.AsTime()
... // make use of t as a time.Time
The AsTime method performs the conversion on a best-effort basis. Timestamps with denormal values (e.g., nanoseconds beyond 0 and 99999999, inclusive) are normalized during the conversion to a time.Time. To manually check for invalid Timestamps per the documented limitations in timestamp.proto, additionally call the CheckValid method:
if err := ts.CheckValid(); err != nil {
... // handle error
}
This is documented in timestamppb package.
Related videos on Youtube
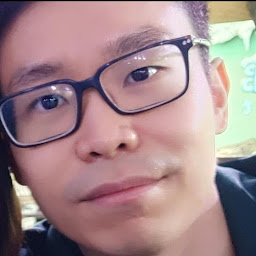
Comments
-
Yuliang Li almost 3 years
I'm using the google time package
github.com/golang/protobuf/ptypes/timestamp
in protobuf message file now.google.protobuf.Timestamp UpdateTime = 9;
But the
UpdateTime
property becomes a pointer*timestamp.Timestamp
in golang struct after protoc compiling, it's not atime.Time
and I can't save these property into Mysql timestamp column.What can I do?
-
Matt Mc about 5 yearsEver figure this out?
-
burx almost 5 yearsptypes already have convenient functions which convert between time.Time and *timestamp.Timestamp. [ godoc.org/github.com/golang/protobuf/ptypes#Timestamp](http://… https://godoc.org/github.com/golang/protobuf/ptypes#TimestampProto
-
infiniteLearner over 3 yearsimport "github.com/golang/protobuf/ptypes" createdObject,err := ptypes.TimestampProto(dbresponse[i].CreatedOn) if err !=nil{ log.Println("Error found:",err) }
-
-
Yuliang Li over 5 yearsSo it need a process to convert the attribute format?
-
Yuliang Li over 5 yearscan protobuf define the attribute
time.Time
type in message? -
colm.anseo almost 5 yearsIn short, yes. A protobuf timestamp has no timezone info. Go's time.Time does. So one would need to add zoneinfo to any message alongside the timestamp. However, since protobuf is mainly used with micro-services & not usually user-facing, maintaining zoneinfo at a micro-service level is most likely not needed.
-
TehSphinX about 3 yearsThis answer is outdated. The function is deprecated.
-
Cosmic Ossifrage almost 3 years@TehSphinX thanks, updated the answer to refer to APIv2 approach.