How can I increment a variable without exceeding a maximum value?
Solution 1
I would just do this. It basically takes the minimum between 100 (the max health) and what the health would be with 15 extra points. It ensures that the user's health does not exceed 100.
public void getHealed() {
health = Math.min(health + 15, 100);
}
To ensure that hitpoints do not drop below zero, you can use a similar function: Math.max
.
public void takeDamage(int damage) {
if(damage > 0) {
health = Math.max(health - damage, 0);
}
}
Solution 2
just add 15 to the health, so:
health += 15;
if(health > 100){
health = 100;
}
However, as bland has noted, sometimes with multi-threading (multiple blocks of code executing at once) having the health go over 100 at any point can cause problems, and changing the health property multiple times can also be bad. In that case, you could do this, as mentioned in other answers.
if(health + 15 > 100) {
health = 100;
} else {
health += 15;
}
Solution 3
You don't need a separate case for each int
above 85
. Just have one else
, so that if the health is already 86
or higher, then just set it directly to 100
.
if(health <= 85)
health += 15;
else
health = 100;
Solution 4
I think an idiomatic, object oriented way of doing this is to have a setHealth
on the Character
class. The implementation of that method will look like this:
public void setHealth(int newValue) {
health = Math.max(0, Math.min(100, newValue))
}
This prevents the health from going below 0 or higher than 100, regardless of what you set it to.
Your getHealed()
implementation can just be this:
public void getHealed() {
setHealth(getHealth() + 15);
}
Whether it makes sense for the Character
to have-a getHealed()
method is an exercise left up to the reader :)
Solution 5
I am just going to offer a more reusable slice of code, its not the smallest but you can use it with any amount so its still worthy to be said
health += amountToHeal;
if (health >= 100)
{
health = 100;
}
You could also change the 100 to a maxHealth variable if you want to add stats to the game your making, so the whole method could be something like this
private int maxHealth = 100;
public void heal(int amountToHeal)
{
health += amountToHeal;
if (health >= maxHealth)
{
health = maxHealth;
}
}
EDIT
For extra information
You could do the same for when the player gets damaged, but you wouldn't need a minHealth because that would be 0 anyways. Doing it this way you would be able to damage and heal any amounts with the same code.
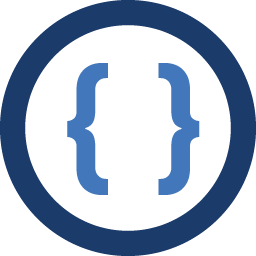
Admin
Updated on June 02, 2022Comments
-
Admin almost 2 years
I am working on a simple video game program for school and I have created a method where the player gets 15 health points if that method is called. I have to keep the health at a max of 100 and with my limited programming ability at this point I am doing something like this.
public void getHealed(){ if(health <= 85) health += 15; else if(health == 86) health += 14; else if(health == 87) health += 13; }// this would continue so that I would never go over 100
I understand my syntax about isn't perfect but my question is, what may be a better way to do it, because I also have to do a similar thing with the damage points and not go below 0.
This is called saturation arithmetic.