How can I kill processes in Android?
29,570
Solution 1
- The message you're getting indicates that your app has crashed. You need to look at its LogCat to find out why. This is documented in the Android Developer Guide.
- Please state your reason for killing all background processes, because I can't think of any appropriate reason to do this. People persist in claiming that "task killers" or "app killers" improve performance, but this attitude ignores the real problem: poorly-written apps. As long as we continue to claim that task killers help, users will continue to use apps that leave unnecessary services, etc. running. Forcing users to use task killers is like dealing with a termite problem by killing one termite at a time as you see them. The real answer is to exterminate all the termites.
In short, anyone who shows you how to kill all background processes is doing you a disservice and the Android community a disservice.
Solution 2
I use this code to kill my own process (app) :
android.os.Process.killProcess(android.os.Process.myPid());
Solution 3
You can use this code:
List<ApplicationInfo> packages;
PackageManager pm;
pm = getPackageManager();
//get a list of installed apps.
packages = pm.getInstalledApplications(0);
ActivityManager mActivityManager = (ActivityManager)context.getSystemService(Context.ACTIVITY_SERVICE);
for (ApplicationInfo packageInfo : packages) {
if((packageInfo.flags & ApplicationInfo.FLAG_SYSTEM)==1)continue;
if(packageInfo.packageName.equals("mypackage")) continue;
mActivityManager.killBackgroundProcesses(packageInfo.packageName);
}
Keep in mind that it's very dangerous to kill apps. If you don't exactly know what you're doing, don't use this code please!
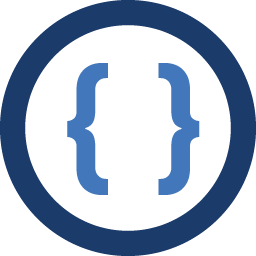
Author by
Admin
Updated on February 18, 2020Comments
-
Admin about 4 years
I have this code below:
package com.example.killall; import android.os.Bundle; import android.app.Activity; import android.view.Menu; import android.view.View; import android.widget.Button; //import android.widget.TextView; import android.app.ActivityManager; public class MainKill extends Activity { private Button BprocessesKill ; //private TextView processesKill; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main_kill); final ActivityManager am=(ActivityManager) getSystemService("SYSTEM_ACTIVITY"); BprocessesKill=(Button) this.findViewById(R.id.BkillProcesses); //processesKill=(TextView) this.findViewById(R.id.killProcesses); BprocessesKill.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View arg0) { am.killBackgroundProcesses(getPackageName()); } }); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.main_kill, menu); return true; } }
All I want to do is simply to press the button and kill all background processes.. the problem I have with this code is that when I am pressing the button it shows me the message : Unfortunately KillAll(that's my app's name) has stopped. What should I change?
-
Joe Malin over 11 yearsDoing this makes sense only as a last resort. If you want me to accept this as good practice, please defend it!
-
Chris Stratton about 10 yearsRegardless of its inadvisability, this is not even an answer to the question asked.
-
Neon Warge about 9 years"In short, anyone who shows you how to kill all background processes is doing you a disservice and the Android community a disservice." +1
-
Dominic Cerisano almost 8 yearsNot all zombies are apps, and the Android community extends beyond app development. Anyone designing distributed systems for mobile is going to need their zombie shotgun while exploring.
-
Dominic Cerisano almost 8 yearsSystem.exit(0) is probably better, since at least some finalizers will get called. Theoretically, it should never be required. You should work within the app lifecycle, and have unit testing in place to cover all the edge cases where the wild things roam.
-
Rombus almost 8 yearsI want to test how an app would react when android kills one of it's background services. Fastest way is knowing how to kill process
-
Gabriel almost 7 yearsGreat, this thread is what google puts first for "android close process by name" and there is no real answer. I'm writing an app for myself which is gonna run only in my phone. The accepted answer is useless. Sometimes SO makes no sense. You have no right to call my needs "a disservice" (note that your lack of capacity for finding out a reason means nothing).
-
Pipin Indrawan over 5 yearssory for updating old thread, kill process is needed in my apps which using JNI lib, because we cannot unload the .so lib, so we kill the process to force destroy the lib. so when the app start again, the lib will be loaded as new.
-
Pipin Indrawan over 5 yearsfor anyone wondering why my app unload the library, because i draw the visual/view in the c++