How can i login in instagram with python requests?
11,976
link = 'https://www.instagram.com/accounts/login/'
login_url = 'https://www.instagram.com/accounts/login/ajax/'
time = int(datetime.now().timestamp())
response = requests.get(link)
csrf = response.cookies['csrftoken']
payload = {
'username': username,
'enc_password': f'#PWD_INSTAGRAM_BROWSER:0:{time}:{password}',
'queryParams': {},
'optIntoOneTap': 'false'
}
login_header = {
"User-Agent": "Mozilla/5.0 (Windows NT 6.1) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/77.0.3865.120 Safari/537.36",
"X-Requested-With": "XMLHttpRequest",
"Referer": "https://www.instagram.com/accounts/login/",
"x-csrftoken": csrf
}
login_response = requests.post(login_url, data=payload, headers=login_header)
json_data = json.loads(login_response.text)
if json_data["authenticated"]:
print("login successful")
cookies = login_response.cookies
cookie_jar = cookies.get_dict()
csrf_token = cookie_jar['csrftoken']
print("csrf_token: ", csrf_token)
session_id = cookie_jar['sessionid']
print("session_id: ", session_id)
else:
print("login failed ", login_response.text)
You can find a complete guide here:
Share a post into your Instagram account using the requests library.
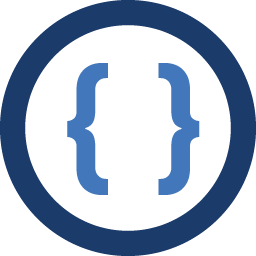
Author by
Admin
Updated on June 23, 2022Comments
-
Admin about 2 years
Hello i am trying to login instagram with python requests library but when i try, instagram turns me "bad requests". İs anyone know how can i solve this problem?
i searched to find a solve for this problem but i didnt find anything. Please help, thanks!
it was working but after some time, it started to turn "bad request"
this is full of my code:
import os import requests import getpass import json import io import time X_SECOND = 60 BASE_URL = "https://www.instagram.com/" LOGIN_URL = BASE_URL + "accounts/login/ajax/" USER_AGENT = "Mozilla/5.0 (Windows NT 10.0; ) Gecko/20100101 Firefox/65.0" CHANGE_URL = "https://www.instagram.com/accounts/web_change_profile_picture/" CHNAGE_DATA = {"Content-Disposition": "form-data", "name": "profile_pic", "filename": "profilepic.jpg", "Content-Type": "image/jpeg"} headers = { "Host": "www.instagram.com", "Accept": "*/*", "Accept-Language": "en-US,en;q=0.5", "Accept-Encoding": "gzip, deflate, br", "Referer": "https://www.instagram.com/accounts/edit/", "X-IG-App-ID": "936619743392459", "X-Requested-With": "XMLHttpRequest", "DNT": "1", "Connection": "keep-alive", } session = requests.Session() session.headers = {'user-agent': USER_AGENT, 'Referer': BASE_URL} def login(): USERNAME = str(input('Username > ')) PASSWD = getpass.getpass('Password > ') resp = session.get(BASE_URL) session.headers.update({'X-CSRFToken': resp.cookies['csrftoken']}) login_data = {'username': USERNAME, 'password': PASSWD} login_resp = session.post(LOGIN_URL, data=login_data, allow_redirects=True) print(login_resp.text) # it turns "bad request" time.sleep(100) if login_resp.json()['authenticated']: print("Login successful") else: print("Login failed!") login() # print(login.json()) session.headers.update({'X-CSRFToken': login_resp.cookies['csrftoken']}) def save(): with open('cookies.txt', 'w+') as f: json.dump(session.cookies.get_dict(), f) with open('headers.txt', 'w+') as f: json.dump(session.headers, f) def load(): with open('cookies.txt', 'r') as f: session.cookies.update(json.load(f)) with open('headers.txt', 'r') as f: session.headers = json.load(f) def change(): session.headers.update(headers) try: print("wow"+str(i)) with open("./data/wow"+str(i)+".png", "rb") as resp: f = resp.read() print("1") p_pic = bytes(f) print("2") p_pic_s = len(f) print("3") session.headers.update({'Content-Length': str(p_pic_s)}) print("4") files = {'profile_pic': p_pic} print("5") r = session.post(CHANGE_URL, files=files, data=CHNAGE_DATA) print("6") if r.json()['changed_profile']: print("Profile picture changed!") else: print("Something went wrong") time.sleep(X_SECOND) except Exception as e: print(e) pass time.sleep(10) if __name__ == "__main__": i = 0 try: load() except: login() save() while True: if i == 12: i = 0 i += 1 change()
AN UPDATE
also the instaloader users are gettin same problem now https://github.com/instaloader/instaloader/issues/615