How can I make net.Read wait for input in golang?
Read is working as expected I think. It sounds like you want the net.Read to work like a channel in Go. This is pretty easy in go just wrap the net.Read in a running goroutine and use select to read from channels a goroutine is really cheap and so is a channel
Example:
ch := make(chan []byte)
eCh := make(chan error)
// Start a goroutine to read from our net connection
go func(ch chan []byte, eCh chan error) {
for {
// try to read the data
data := make([]byte, 512)
_,err := conn.Read(data)
if err != nil {
// send an error if it's encountered
eCh<- err
return
}
// send data if we read some.
ch<- data
}
}(ch, eCh)
ticker := time.Tick(time.Second)
// continuously read from the connection
for {
select {
// This case means we recieved data on the connection
case data := <-ch:
// Do something with the data
// This case means we got an error and the goroutine has finished
case err := <-eCh:
// handle our error then exit for loop
break;
// This will timeout on the read.
case <-ticker:
// do nothing? this is just so we can time out if we need to.
// you probably don't even need to have this here unless you want
// do something specifically on the timeout.
}
}
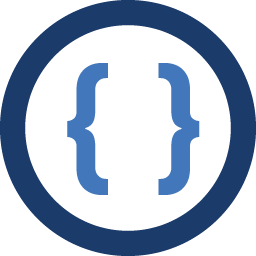
Admin
Updated on June 16, 2022Comments
-
Admin almost 2 years
So I'm making a server for my elevator in Go, and I'm running the function "handler" as a goroutine with a TCP-connection. I want it to read from a connection, and if no signal is detected within a certain timespan I want it to return an error.
func handler(conn net.Conn){ conn.SetReadTimeout(5e9) for{ data := make([]byte, 512) _,err := conn.Read(data) } }
As long as I have a client sending stuff over the connection it seems to be working fine, but as soon as the client stops sending the net.Read function returns the error EOF and starts looping with no delay whatsoever.
This might be how Read is supposed to work, but could someone suggest another way to handle the problem without having to close and open the connection every time I want to read something?
-
Dave C almost 9 yearsThe documentation explicitly warns against using
time.Tick
this way. -
nfirvine over 8 yearsAlso, I'd say a value much smaller than a second would be more appropriate.