How can I multiply a string in the C language?
Solution 1
So you need 2 loops to do this. One for iterating through the characters you want to print on a line, one to iterate through the entire height (number of lines).
So what we want to do is:
- Go through each line from 1 up to and including the height.
- For each line, output as many #'s as the current line number
e.g.
int lineno;
int height = GetInt();
...
for (lineno = 1; lineno <= height; lineno++) {
int column;
for (column = 0; column < lineno; column++) {
putchar('#');
}
putchar('\n');
}
This will be a left adjusted tree. I'll leave it up to you to right adjust it, i.e. print spaces in front of the '#', or start by printing 2 #'s instead of 1.
Solution 2
You don't multiply a string, you simply use a loop and output the character repeatedly.
int j;
for (j = 0; j < (1 + height); ++j) {
printf ("#"); /* Or putchar('#') */
}
printf ("\n");
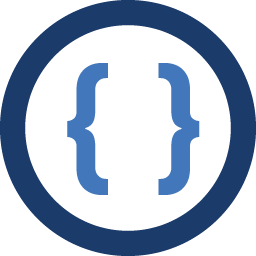
Admin
Updated on June 26, 2022Comments
-
Admin about 2 years
I want to "multiply" a string by an
int
variable that the user inputs.#include <cs50.h> #include <stdio.h> // Height < 24 string block = "#"; string output; int main(void) { printf("Height: "); int height = GetInt(); while (true) { if (height < 24 && height > 0) { output = "#" + block * height; printf("%s\n", output); break; } else { printf("Height: "); height = GetInt(); } } return 0; }
Using the
Height
variable I want to multiply the string variableblock (#)
byHeight
, and add that to another"#"
.I tried implementing it in the only way I could think of it making sense however it doesnt seem the syntax is right.
I've looked over StackOverflow on this subject and can only find C# and C++ topics with this question in mind.
EDIT: After being printed the output should look like this:
## ### #### ##### ###### ####### ######## #########
And the lines of "#" being outputted depends on the Height variable that the user inputs. Say the user inputs a height of "5":
Height: 5 ## ### #### ##### ######
Should be output.
-
Peter - Reinstate Monica over 8 yearsThis will segfault with a probability of about 1-1E-6.