How can I obtain a list of currently running applications?
You can use the Process.GetProcesses
method to provide information about all of the processes that are currently running on your computer.
However, this shows all running processes, including ones that are not necessarily shown on the taskbar. So what you'll need to do is filter out those processes that have an empty MainWindowTitle
.The above-linked documentation explains why this works:
A process has a main window associated with it only if the process has a graphical interface. If the associated process does not have a main window (so that MainWindowHandle is zero), MainWindowTitle is an empty string ("").
So, you could use something like the following code, which will print out (to a console window) a list of all currently running applications that are visible on your taskbar:
Process[] processes = Process.GetProcesses();
foreach (var proc in processes)
{
if (!string.IsNullOrEmpty(proc.MainWindowTitle))
Console.WriteLine(proc.MainWindowTitle);
}
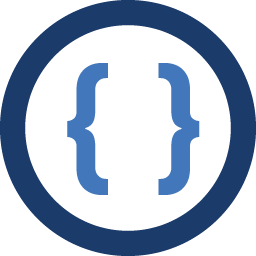
Admin
Updated on June 18, 2022Comments
-
Admin almost 2 years
I want to programmatically obtain a list of running (desktop) applications, and then I want to display this list to the user. It should be something similar to the application list displayed in the Windows Task Manager.
How can I create this in C#? Specifically, I need a way to obtain that list of currently running applications.
-
Ed. almost 12 yearsI apologize for this 2-years-later comment, but I'm looking for a similar solution, and wanted to mention that the above solution does not work (AFAIK) in cases like WORD or ACRO-READER that may have more than one document opened on the desktop. In that case, it appears that only the topmost window is listed as an application using the above method. If I'm wrong I apologize, but assuming I'm right, I'd like to know how to list all windows, even if they are from the same underlying process (like acrord or word).
-
Cody Gray almost 12 years@Ed. Yeah, this enumerates processes, but those applications are only running a single process. It's inconsequential that the process manages multiple top-level windows. You can enumerate top-level windows using the
EnumWindows
function. Call this function and specify a pointer to a callback function. The callback function will be called once for each top-level window on the screen. -
Alok Rajasukumaran about 4 yearsif we have 2 display monitors, how to know which monitor this process is running?
-
Cody Gray about 4 yearsProcesses do not have monitor affinity, @Alok. Some processors don't display any user interface whatsoever, and thus don't appear on a monitor. Other processes may display multiple windows, and those windows may appear on several different monitors. You need to choose a window, and then see which monitor that window is on. In Win32, you'd use the
MonitorFromWindow
function, passing in a window handle (HWND
) and getting a monitor handle (HMONITOR
). You can P/Invoke that in .NET, or you can useSystem.Windows.Forms.Screen.FromPoint
directly from WinForms.