How can I pass an object to a JSP tag?
Solution 1
A slightly different question that I looked for here: "How do you pass an object to a tag file?"
Answer: Use the "type" attribute of the attribute directive:
<%@ attribute name="field"
required="true"
type="com.mycompany.MyClass" %>
The type defaults to java.lang.String, so without it you'll get an error if you try to access object fields saying that it can't find the field from type String.
Solution 2
<jsp:useBean id="myObject" class="java.lang.Object" scope="page" />
<wf:my-tag obj="${myObject}" />
Its not encouraged to use Scriptlets in JSP page. It kills the purpose of a template language.
Solution 3
The original syntax was to reuse '<%= %>'
So
<wf:my-tag obj="<%= myObject %>" />
See this part of the Sun Tag Library Tutorial for an example
Solution 4
For me expression language works only if I make that variable accessible, by putting it for example in page context.
<% Object myObject = new Object();
pageContext.setAttribute("myObject", myObject);
%>
<wf:my-tag obj="${myObject}" />
Otherwise tas receives null.
And <wf:my-tag obj="<%= myObject %>" />
works with no additional effort. Also <%=%> gives jsp compile-time type validation, while El is validated only in runtime.
Solution 5
You can use "<%= %>" to get the object value directly in your tag :
<wf:my-tag obj="<%= myObject %>"/>
and to get the value of any variable within that object you can get that using "obj.parameter" like:
<wf:my-tag obj="<%= myObject.variableName %>"/>
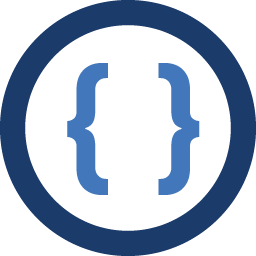
Admin
Updated on December 11, 2020Comments
-
Admin over 3 years
I have a JSP page that contains a scriplet where I instantiate an object. I would like to pass that object to the JSP tag without using any cache.
For example I would like to accomplish this:
<%@ taglib prefix="wf" uri="JspCustomTag" %> <% Object myObject = new Object(); %> <wf:my-tag obj=myObject />
I'm trying to avoid directly interacting with any of the caches (page, session, servletcontext), I would rather have my tag handle that.