How can I pass data from express server to react views?
I think your best option is to indeed make some kind of network request from the client. If you aim to keep the app simple and do not want a State Management library (e.g. Redux), you could do something like
class IndexPage extends React.Component {
constructor(props) {
super(props);
this.state = {
posts: []
};
}
componentDidMount() {
fetch('/') // or whatever URL you want
.then((response) => response.json())
.then((posts) => this.setState({
posts: posts,
});
}
render() {
return (
<div>
<Posts posts={this.state.posts} />
</div>
);
}
}
In your response
there should be a JSON representation of the posts collection.
Also note the render
method and accessing the posts
.
For more on Fetch API see MDN (please also note that you will need a polyfill for older browsers for it).
EDIT: For socket.io I'd store the instance of it somewhere and pass it as a prop to the component. Then you can do something like
class IndexPage extends React.Component {
...
componentDidMount() {
this.props.socket.on('postReceived', this.handleNewPost);
}
handleNewPost = (post) => {
this.setState({
posts: [
...this.state.posts,
post,
],
});
}
...
}
The server-side part is similar, see for example Socket.io Chat example.
Related videos on Youtube
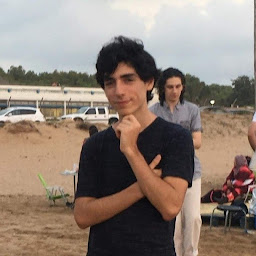
Santiago Aranguri
Updated on June 04, 2022Comments
-
Santiago Aranguri almost 2 years
I have a simple express server with a connection to a orientdb database. I need to pass information from express to react views. For example, in express I have:
router.get('/', function(req, res, next) { Vertex.getFromClass('Post').then( function (posts) { res.render('index', { title: 'express' }); } ); });
So, in this example, I need to have in my react index component, the
posts
variable to set the state of the componenent. (I'm using react only in the frontend, not server-side)class IndexPage extends React.Component { constructor(props) { super(props); this.state = { posts: [] }; } render() { return ( <div> <Posts posts={posts} /> </div> ); } }
How can I get the posts in react from express?
I found that maybe I can do an ajax request from react, but I think that that isn't the best way.
If I need to get that posts in a real time way, using socket.io for example, what are the differences?
PD: In express I have the possibility to use some template engine like handlebars or hogan. Can this template engines help in this topic?
Thanks!!!
-
PapaDiHatti over 6 yearsThere is no server side react, it can only be used in frontend
-
-
Santiago Aranguri over 7 yearsThanks! And if I need to do this in a real-time way, there is some function like fetch in socket.io? Or is a totally different way in real-time? What about the server, is the same
-
Santiago Aranguri over 7 yearsWhat about the server, is the same if I fetch the data real time, or there are many changes?
-
Dan Homola over 7 yearsI don't have much experience in real-time web, but I don't think it really matters to React. All you need to do is to call
setState
whenever new data arrive (there will be some callback from socket.io). The server-side would use socket.io to push JSON to the client. It is best to find some tutorial for that, I'm sure there are plenty.