How can I pop to specific screen in flutter
5,419
Solution 1
If you didn't define any route in the MaterialApp then you need to define at the time of push.
Navigator.of(context).push(
MaterialPageRoute(builder: (_) {
return SecondPage();
},
settings: RouteSettings(name: 'SecondPage',),
));
You need to define the same route name
Navigator.of(context).popUntil((route){
return route.settings.name == 'SecondPage';
});
Solution 2
I believe that would be the Navigator.popUntil Method.
You need to have your routes defined in your materialApp for Navigator to recognise it. For the code below to work "/home" needs to be defined in my MaterialApp.
Navigator.popUntil(context, ModalRoute.withName('/home'));
Solution 3
try pushNamedAndRemoveUntil
,
Navigator.of(context).pushNamedAndRemoveUntil(
'/BottomNavigation', (Route<dynamic> route) => false);
For in depth reading follow this
Solution 4
If you don't want to use named route then simply use-- pushAndRemoveUntil
Example--
Navigator.pushAndRemoveUntil(context, MaterialPageRoute(
builder: (context) => MyHomePage()), (route) => false);
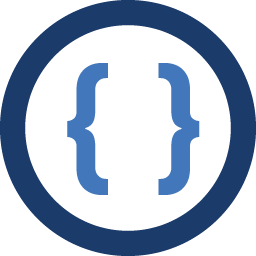
Author by
Admin
Updated on December 16, 2022Comments
-
Admin over 1 year
I pushed four screens ScreenOne >> ScreenTwo >> ScreenThree >> ScreenFour
and I'm at ScreenFour now I want to pop back to ScreenTwo
In Swift it works like this::
if let viewControllers: [UIViewController] = self.navigationController!.viewControllers { for vc in viewControllers { if (vc is SecondViewController) { self.navigationController!.popToViewController(vc, animated: true) } } }
how i perform this operation in flutter.
Please give a solution, Thank you.