How can I prevent my Android app/service from being "killed" from a task manager?
Solution 1
You can use API method: startForeground()
. Here is the explanation of it:
A started service can use the startForeground(int, Notification) API to put the service in a foreground state, where the system considers it to be something the user is actively aware of and thus not a candidate for killing when low on memory. (It is still theoretically possible for the service to be killed under extreme memory pressure from the current foreground application, but in practice this should not be a concern.)
Here you can find an example how to use this.
As for the question, you cannot prevent a service from being killed. It can be killed by the system. Even system services can be killed. If this happens they are restarted. You may use the same approach.
Solution 2
You can write a helper app to receive android broadcast "android.intent.action.PACKAGE_RESTARTED",when your app got killed,your helper will receive that broadcast and you can restart your app or whatever.
That's how 'Smart App Protector Free' do.
The bad thing is users must install two apps instead of one.
Solution 3
There isn't a way to prevent this directly, without a rooted device. The SDK helpfully prevents these kinds of issues.
You can do the "truly evil" trick and have two services in two application. Each service monitors the other, and restarts it if it stops. This is kludgy, but in most cases even the fastest fingered kid couldn't kill both applications.
Solution 4
For anyone who is still searching for an answer - this one may be correct:
you can not: make a service unkillable, if running on low Memory the System will always kill your service. BUT
you can: Tell the System to restart your service when it is killed. Look at this piece of code:
public static final int START_REDELIVER_INTENT
Added in API level 5
Constant to return from onStartCommand(Intent, int, int)
:
if this service's process is killed while it is started (after returning from onStartCommand(Intent, int, int))
, then it will be scheduled for a restart and the last delivered Intent re-delivered to it again via onStartCommand(Intent, int, int)
.
This Intent will remain scheduled for redelivery until the service calls stopSelf(int)
with the start ID provided to onStartCommand(Intent, int, int)
. The service will not receive an onStartCommand(Intent, int, int)
call with a null Intent because it will only be re-started if it is not finished processing all Intents sent to it (and any such pending events will be delivered at the point of restart).
Constant Value: 3 (0x00000003)
Solution 5
If you have system level permissions use persistent:true
via manifest permissions.
https://developer.android.com/guide/topics/manifest/application-element
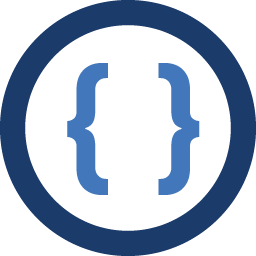
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
It is very important that my service stay running until someone with a password stops the service from my UI screen. My app runs great but it is designed to be turned on/off by parents (with a password) on their kids phones. I have managed to make everything work but the problem I'm having is that if the kid uses a task manager to kill my service then my app is useless. I would be grateful to anyone who knows a way to either
1) monitor the service and start it back up automatically if its "killed" or 2) prevent someone from being able to kill it except from the activity (administration screen) that launched the service. Or both?
I'm sorry if I'm not very clear in describing the problem, I'm a beginner. I've made great progress so far but I am stuck at this last hurdle.
-
C. K. Young almost 14 yearsBut a program can! Any program that can execute the equivalent of
kill -KILL -1
can cause all your instances to die simultaneously. -
rayman over 13 yearsIf you would have system privileges, how would you sign your app in a way of hiding it or un-kill able from users? is there any specific permission you have to set?
-
xandy almost 13 yearsI just installed an app called Smart App Protector Free, it ask you if you wanna install a helper than prevent it being killed. It works and I wonder how it is. I use the System Panel to kill everything (including system process) but the protector still come back. Even more, I can't see trace of the helper running, not in the System Panel, and even I ps in the device console (thru adb), I still can't see that helper exists. Any hints?
-
CopsOnRoad over 5 yearsIt should be
START_STICKY
-
Slawa over 5 yearsOnly works for system processes. User-installed processes have this forced to false.
-
Ofek Regev about 5 yearswill it work with an application which has a system permissions on a rooted device?
-
JoshuaTree about 5 yearsI am not sure, I have never rooted a device before, but I should. Anyways, I think with a rooted device you could probably do anything :)