How can I print a euro (€) symbol in Python?
Solution 1
You can use Unicode Character Name
\N{name}
is great way to print unicode character symbol.
Here is sample run....
>>> print "Please pay %s"%(u"\N{euro sign}")
Please pay €
>>> print "Please pay %s"%(u"\N{dollar sign}")
Please pay $
>>> print "Please pay %s"%(u"\N{rupee sign}")
Please pay ₨
>>> print "Please pay %s"%(u"\N{pound sign}")
Please pay £
Solution 2
Here are four ways of printing a string with the Euro symbol in Python 3.x, in increasing order of obscurity.
1. Direct input
Use your keyboard to enter the symbol, or copy and paste it from somewhere else:
mytext = "Please pay €35."
print(mytext)
2. Use Unicode glyph number
Look up the Unicode glyph number, for example from the very useful page http://www.fileformat.info/info/unicode/, and use that code in your string:
mytext = "Please pay \u20ac35."
print(mytext)
3. Use glyph name
You can use lookup()
from the unicodedata
module to access Unicode glyphs by name. Again http://www.fileformat.info/info/unicode/ will help you find the glyph name:
import unicodedata
mytext = "Please pay {}35.".format(unicodedata.lookup("EURO SIGN"))
print(mytext)
4. Use Windows-1252 codepage
If you really want to use the \x80
byte code, you can do that, because it represents the Euro symbol in the Windows-1252 codepage. What you need to do is first create a byte string containing that byte code, and then decoding that byte string so that the byte code is translated to the Euro symbol entry in the Windows-1252 codepage:
mytext = b"Please pay \x8035.".decode("windows-1252")
print(mytext)
Solution 3
You can use this:
mytext = 'Please pay \u20ac.'
print(mytext)
... based on Unicode Character 'EURO SIGN'.
But if the character can be represented in the script's character encoding, then there is no reason why you shouldn't write:
mytext = 'Please pay €.'
Solution 4
Euro is encoded as 80h
(0x80
) in the windows-1252
charset and you must tell Python that:
# -*- coding: utf-8 -*-
mytext = b'Please pay \x8035.'.decode('windows-1252')
print(mytext)
You could also write the real euro character directly in the source code or use its representation, as others said, using the correct encoding (utf-8
):
# -*- coding: utf-8 -*-
mytext = u'Please pay \u20ac35.'
print(mytext)
... or ...
# -*- coding: utf-8 -*-
mytext = 'Please pay €35.'
print(mytext)
This code works both in Python 2 and 3.
Solution 5
From http://www.python-forum.org/viewtopic.php?f=6&t=13995 :
This is behavior of python 2,Python 3 does not do this.
Python 2.7:
>>> s = "€"
>>> s
'\xe2\x82\xac'
>>> print s
€
>>> s = u"€"
>>> s
u'\u20ac'
>>> print s
€
>>> print('\xe2\x82\xac'.decode('utf8'))
€
Python 3.4
>>> s = '€'
>>> s
'€'
>>> print(s)
€
>>>
>>> print(repr(s))
'€'
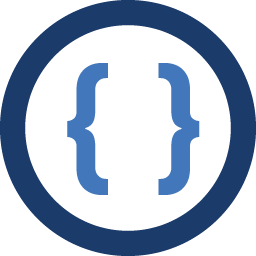
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm teaching myself Python using the command-line interpreter (v3.5 for Windows).
All I want to do is output some text that includes the euro (€) symbol which I understand to be code 80h (128 dec).
#! # -*- coding: utf-8 -*- mytext = 'Please pay \x8035.' print(mytext)
It falls over on the last line:
UnicodeEncodeError: 'charmap' codec can't encode character '\x80' in position 11: character maps to <undefined>
I've done lots of googling (re encodings etc) and I've a rough idea why the print command fails. Tinkering with the above code shows that ASCII codes up to \x7f work fine, as one might expect.
But I can't figure out how to display the €, and I'm finding the information on encodings overwhelming and impenetrable. (Remember I'm just a noob!)
- I've tried prefixing the text string with u to create a unicode string in the first place.
- I've tried creating an intermediate object outputtext = mytext.encode('utf-8') but outputting this with print expands the string into an even more cryptic form: b'Please pay \xc2\x8035.'
- I've tried to find a different function instead of print to output this intermediate string, but nothing has worked yet.
Please can someone show me some code that just works, so I can study it and work backwards from there. Thanks!