How can I recieve array using Yii ActiveRecord
Solution 1
Duplicate: Yii - How to get a values array from an Active Record
use CHtml::listData
(see http://www.yiiframework.com/wiki/48/by-example-chtml/#hh3 )
$users = User::model()->findAll();
$usersArr = CHtml::listData( $users, 'id' , 'city');
print_r( $usersArr );
It will give you array id => city
Array {
2 => 'Paris',
102 => 'Riga',
// ...
}
Solution 2
Actually I have dealt with the same problem, I would like to use the ActiveRecord instead of CDBCommand. I have answered on the similar question before
$words = Word::model()->findAll();
$data=array_map(create_function('$m','return $m->getAttributes();'),$words);
var_dump($data);
Solution 3
An active record, by it's name, will return a whole record for each row in your database, so the best way to obtain just one field from each row would be using the querybuilder with something like what you've got above.
If you really wanted to use AR and just want the name in an array then something like this might work:
$names = array();
$users = users::model()->findAllByAttributes(array('city'=>'Paris'));
foreach(array_keys($users) as $key)
{
$names[] = $users[$key]->name;
}
Although that's a lot of overhead to pull just the name if you're not using any other details from the AR search.
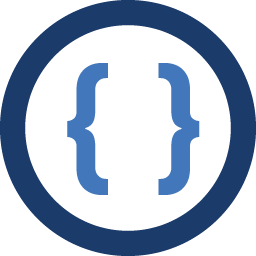
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
Consider this query and its result:
Yii::app()->db->createCommand( "SELECT name FROM users WHERE city='Paris'" )->queryColumn();
Result:
Array ( [0] => John Kerry [1] => Marry White [2] => Mike Jain )
Any suggestions on how to build this query with
ActiveRecord
? It is necessary to receive the array. -
Maykonn over 10 yearsKludge! See the answer of @Telvin Nguyen. But better is use an anonymous function to extract result from findAll as a array.
-
Steven about 9 yearsdoesn't ActiveRecord return objects?
array_map
expects parameter 2 to be an array - but since $words is an object, we get an error. (?) -
Telvin Nguyen about 9 years@Steven CActiveRecord comprises the findall method return the array of records found. An empty array is returned if none is found. In my example, the $words variable always be an array.