How can I remove ".0" of float numbers?
Solution 1
Here's a function to format your numbers the way you want them:
def formatNumber(num):
if num % 1 == 0:
return int(num)
else:
return num
For example:
formatNumber(3.11111)
returns
3.11111
formatNumber(3.0)
returns
3
Solution 2
You can combine the 2 previous answers :
formatNumber = lambda n: n if n%1 else int(n)
>>>formatNumber(5)
5
>>>formatNumber(5.23)
5.23
>>>formatNumber(6.0)
6
Solution 3
you can use string formatting
>>> "%g" % 1.1
'1.1'
>>> "%g" % 1.0
'1'
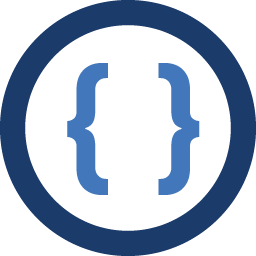
Admin
Updated on July 21, 2022Comments
-
Admin almost 2 years
Say I have a float number. If it is an integer (e.g. 1.0, 9.0, 36.0), I want to remove the ".0 (the decimal point and zero)" and write to stdout. For example, the result will be 1, 9, 36. If the float number is a rational number such as 2.5335 and 95.5893, I want the output to be as it was inputted, meaning 2.5335 and 95.5893. Is there a smart way to do this?
Only whether the number has .0 or other digits at decimal places does matter. I can say my question is this: how can I know whether or not a float number is actually an integer?
I want to use this to decide directory names. For example, if I input 9.0 and 2.45, make two directories, dir9 and dir2.45.
-
Vicki B over 4 yearsunfortunately, int(3.5) also returns 3 which the OP specifically does not want to have happen.
-
kemot25 over 3 yearsIt's possible to define
formatNumber = lambda n: int(n) if isinstance(n,float) and n.is_integer() else n