How can I rotate an image using Java/Swing and then set its origin to 0,0?
Solution 1
Use the g2d.transform() to shift the image back where it's needed. I'm just imagining what the calculation might be but I think something like:
int diff = ( image.getWidth() - image.getHeight() ) / 2;
g2.transform( -diff, diff );
BTW, you may have a problem with the label reporting its preferred size - you might need to override getPreferredSize() and account for swapping the images width and height if rotated.
Solution 2
AffineTransform trans = new AffineTransform(0.0, 1.0, -1.0, 0.0, image.getHeight(), 0.0);
g2d.transform(trans);
g2d.drawImage(image, 0, 0, image.getWidth(), image.getHeight(), null);
Solution 3
The following function will rotate a buffered image that comes in indeterminate if it is a perfect square or not.
public BufferedImage rotate(BufferedImage image)
{
/*
* Affline transform only works with perfect squares. The following
* code is used to take any rectangle image and rotate it correctly.
* To do this it chooses a center point that is half the greater
* length and tricks the library to think the image is a perfect
* square, then it does the rotation and tells the library where
* to find the correct top left point. The special cases in each
* orientation happen when the extra image that doesn't exist is
* either on the left or on top of the image being rotated. In
* both cases the point is adjusted by the difference in the
* longer side and the shorter side to get the point at the
* correct top left corner of the image. NOTE: the x and y
* axes also rotate with the image so where width > height
* the adjustments always happen on the y axis and where
* the height > width the adjustments happen on the x axis.
*
*/
AffineTransform xform = new AffineTransform();
if (image.getWidth() > image.getHeight())
{
xform.setToTranslation(0.5 * image.getWidth(), 0.5 * image.getWidth());
xform.rotate(_theta);
int diff = image.getWidth() - image.getHeight();
switch (_thetaInDegrees)
{
case 90:
xform.translate(-0.5 * image.getWidth(), -0.5 * image.getWidth() + diff);
break;
case 180:
xform.translate(-0.5 * image.getWidth(), -0.5 * image.getWidth() + diff);
break;
default:
xform.translate(-0.5 * image.getWidth(), -0.5 * image.getWidth());
break;
}
}
else if (image.getHeight() > image.getWidth())
{
xform.setToTranslation(0.5 * image.getHeight(), 0.5 * image.getHeight());
xform.rotate(_theta);
int diff = image.getHeight() - image.getWidth();
switch (_thetaInDegrees)
{
case 180:
xform.translate(-0.5 * image.getHeight() + diff, -0.5 * image.getHeight());
break;
case 270:
xform.translate(-0.5 * image.getHeight() + diff, -0.5 * image.getHeight());
break;
default:
xform.translate(-0.5 * image.getHeight(), -0.5 * image.getHeight());
break;
}
}
else
{
xform.setToTranslation(0.5 * image.getWidth(), 0.5 * image.getHeight());
xform.rotate(_theta);
xform.translate(-0.5 * image.getHeight(), -0.5 * image.getWidth());
}
AffineTransformOp op = new AffineTransformOp(xform, AffineTransformOp.TYPE_BILINEAR);
BufferedImage newImage =new BufferedImage(image.getHeight(), image.getWidth(), image.getType());
return op.filter(image, newImage);
}
Solution 4
The Rotated Icon class might be what you are looking for.
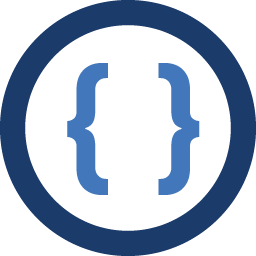
Admin
Updated on June 23, 2022Comments
-
Admin almost 2 years
I'm able to rotate an image that has been added to a JLabel. The only problem is that if the height and width are not equal, the rotated image will no longer appear at the JLabel's origin (0,0).
Here's what I'm doing. I've also tried using AffineTransform and rotating the image itself, but with the same results.
Graphics2D g2d = (Graphics2D)g; g2d.rotate(Math.toRadians(90), image.getWidth()/2, image.getHeight()/2); super.paintComponent(g2d);
If I have an image whose width is greater than its height, rotating that image using this method and then painting it will result in the image being painted vertically above the point 0,0, and horizontally to the right of the point 0,0.