How can I save a JSON response to a file that would be accessible from within a local HTML file loaded inside UIWebWiew
After working with the idea some more here is what I came up with.
When the application is installed there is a default data file in the package that I copy to the Documents folder. When the application didFinishLaunchingWithOptions
runs I call the following method:
- (void)writeJsonToFile
{
//applications Documents dirctory path
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
//live json data url
NSString *stringURL = @"http://path-to-live-file.json";
NSURL *url = [NSURL URLWithString:stringURL];
NSData *urlData = [NSData dataWithContentsOfURL:url];
//attempt to download live data
if (urlData)
{
NSString *filePath = [NSString stringWithFormat:@"%@/%@", documentsDirectory,@"data.json"];
[urlData writeToFile:filePath atomically:YES];
}
//copy data from initial package into the applications Documents folder
else
{
//file to write to
NSString *filePath = [NSString stringWithFormat:@"%@/%@", documentsDirectory,@"data.json"];
//file to copy from
NSString *json = [ [NSBundle mainBundle] pathForResource:@"data" ofType:@"json" inDirectory:@"html/data" ];
NSData *jsonData = [NSData dataWithContentsOfFile:json options:kNilOptions error:nil];
//write file to device
[jsonData writeToFile:filePath atomically:YES];
}
}
Then throughout the application when I need to reference the data, I use the saved file.
//application Documents dirctory path
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
NSError *jsonError = nil;
NSString *jsonFilePath = [NSString stringWithFormat:@"%@/%@", documentsDirectory,@"data.json"];
NSData *jsonData = [NSData dataWithContentsOfFile:jsonFilePath options:kNilOptions error:&jsonError ];
To reference the json file in my JS code, I added a URL parameter for "src" and passed the file path to the Applications Documents folder.
request.open('GET', src, false);
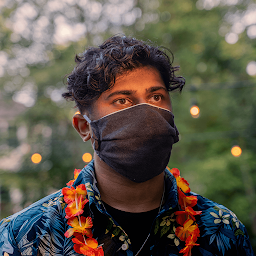
Ajan Patel
Updated on June 30, 2022Comments
-
Ajan Patel almost 2 years
I am receiving a JSON response and am currenlty able to make use of the data within my app.
I would like to save this response to a file so I could reference within an JS file located inside my project. I have already requested this data once when the application is launched so why not save it to a file and reference that throughout so only one call is needed for the data.
The HTML files for my UIWebView are imported into my Xcode project using the "create folder reference" option and the path to my JS file is
html->js->app.js
I want to save the response as
data.json
somewhere on the device and then reference inside my js file like thisrequest.open('GET', 'file-path-to-saved-json.data-file', false);
How can I achieve this?