How can I set a default profile for the Firefox driver in Selenium Webdriver 3?
Solution 1
As you are using Selenium 3.14.0 as per the FirefoxDriver Class the valid constructors are:
FirefoxDriver()
FirefoxDriver(FirefoxOptions options)
FirefoxDriver(GeckoDriverService service)
FirefoxDriver(GeckoDriverService service, FirefoxOptions options)
FirefoxDriver(XpiDriverService service)
FirefoxDriver(XpiDriverService service, FirefoxOptions options)
So, as per your code attempts the following is not a valid option to invoke FirefoxDriver()
WebDriver driver = new FirefoxDriver(profile);
Solution
To invoke invoke FirefoxDriver()
with the default profile you need to use the setProfile(profile)
method to set the FirefoxProfile through an instance of FirefoxOptions()
and you can use the following code block:
-
Code Block:
import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.firefox.FirefoxOptions; import org.openqa.selenium.firefox.FirefoxProfile; import org.openqa.selenium.firefox.ProfilesIni; import org.testng.annotations.Test; public class A_FirefoxProfile { @Test public void seleniumFirefox() { System.setProperty("webdriver.gecko.driver", "C:/Utility/BrowserDrivers/geckodriver.exe"); ProfilesIni profileIni = new ProfilesIni(); FirefoxProfile profile = profileIni.getProfile("default"); FirefoxOptions options = new FirefoxOptions(); options.setProfile(profile); WebDriver driver = new FirefoxDriver(options); driver.get("http://www.google.com"); System.out.println(driver.getTitle()); } }
-
Console Output:
[RemoteTestNG] detected TestNG version 6.14.2 1537775040906 geckodriver INFO geckodriver 0.20.1 1537775040923 geckodriver INFO Listening on 127.0.0.1:28133 Sep 24, 2018 1:14:30 PM org.openqa.selenium.remote.ProtocolHandshake createSession INFO: Detected dialect: W3C Google PASSED: seleniumFirefox =============================================== Default test Tests run: 1, Failures: 0, Skips: 0 =============================================== =============================================== Default suite Total tests run: 1, Failures: 0, Skips: 0 ===============================================
Solution 2
move your setup to @BeforeClass
I'm using this setup, works just fine:
@BeforeClass
public static void setUpClass() {
FirefoxOptions options = new FirefoxOptions();
ProfilesIni allProfiles = new ProfilesIni();
FirefoxProfile selenium_profile = allProfiles.getProfile("selenium_profile");
options.setProfile(selenium_profile);
options.setBinary("C:\\Program Files (x86)\\Mozilla Firefox\\firefox.exe");
System.setProperty("webdriver.gecko.driver", "C:\\Users\\pburgr\\Desktop\\geckodriver-v0.20.0-win64\\geckodriver.exe");
driver = new FirefoxDriver(options);
driver.manage().window().maximize();}
just change paths and profile designation.
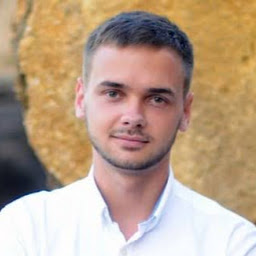
Vladyslav Kuhivchak
Updated on June 19, 2022Comments
-
Vladyslav Kuhivchak almost 2 years
I can't set a default profile for Firefox in Selenium Webdriver 3 because there is no such constructor in the
FirefoxDriver
class.import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.firefox.FirefoxProfile; import org.openqa.selenium.firefox.ProfilesIni; import org.testng.annotations.BeforeMethod; import org.testng.annotations.Test; public class SeleniumStartTest { @Test public void seleniumFirefox() { System.setProperty("webdriver.gecko.driver", "C:\\Users\\FirefoxDriver\\geckodriver.exe"); ProfilesIni profileIni = new ProfilesIni(); FirefoxProfile profile = profileIni.getProfile("default"); WebDriver driver = new FirefoxDriver(profile); driver.get("http://www.google.com"); } }
Compile error in Java code: Java Code
Maven
pom.xml
dependencies: Selenium 3.14.0Firefox version: Firefox version 62.0.2