How can I solve this MATLAB "Matrix dimensions must agree" error?
UPDATE:
OK, now that you have confirmed that your variables x2
and y1
contain different numbers of elements, you have a couple of solutions to choose from:
-
For each variable, you can create a set number of values over the respective ranges using the function LINSPACE. For example:
x2 = linspace(0,5,101); %# 101 values spanning the range 0 to 5 y1 = linspace(-5,5,101); %# 101 values spanning the range -5 to 5
However, when you compute the result
f32
(which will also be a 101-element array), it will only be evaluated at the respective pairs of values inx2
andy1
(e.g.x2(1)
andy1(1)
,x2(50)
andy1(50)
, etc.). -
If you would rather evaluate
f32
at every unique pair of points over the ranges ofx2
andy1
, you should instead use the function MESHGRID to generate your values. This will also allow you to have a different numbers of points over the ranges forx2
andy1
:[x2,y1] = meshgrid(0:0.1:5,-5:0.1:5);
The above will create
x2
andy1
as 101-by-51 arrays such thatf32
will also be a 101-by-51 array evaluated at all the points over the given ranges of values.
Previous answer:
The first thing to test is if all the variables you are putting into the equation are the same size or scalar values, which they would have to be since you are using element-wise operators like .^
and .*
. For the first equation, see what output you get when you do this:
size(x2)
size(y1)
If they give the same result, or either is [1 1]
, then that's not your problem.
The next thing to check is whether or not you have shadowed the EXP function by creating a variable by the name exp
. If you're running the code as a script in the command window, type whos
and see if a variable named exp
shows up. If it does, you need to delete or rename it so that you can use the function EXP.
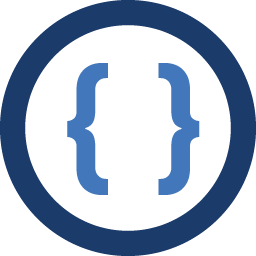
Admin
Updated on October 30, 2020Comments
-
Admin over 3 years
I am typing some code for a class but every time I run the function I get the same error:
??? Error using ==> plus Matrix dimensions must agree. Error in ==> Test at 6 f32=3.*exp((-x2.^2-y1.^2)./3);
I know that the problem is a simple index error, but I can't seem to find it anywhere. Can somebody help me?
Also I'm having the same problem with the following line of code:
f34=(exp(-0.3./x2))./(log(y2).*sqrt(x2));
EDIT #1:
x2
is defined as0:0.1:5
andy1
is defined as-5:0.1:5
, but that is what I have been assigned to define them as. And I know exp is not a function because I have used it elsewhere in my file.EDIT #2:
OK. So if I can't use my current x and y is there anyway I can define them to keep them on those bounds while still making them the same size?