How can I store data in RAM memory using PHP?
Solution 1
APC?
It works differents from memcached; in memcached you can access the data from various languages (c, python, etc..) while APC works only for PHP.
EDIT are you sure that APC is installed correctly?
Did you add extension=apc.so
in your php.ini? And to restart apache (im assuming youre on a lamp server with apache2)? What does you phpinfo();
say about APC?
This is a simply test that work perfectly for me:
<?php
/*
* page.php
* Store the variable for 30 seconds,
* see http://it.php.net/manual/en/function.apc-add.php
* */
if(apc_add('foo', 'bar', 30)){
header('Location: page2.php');
}else{
die("Cant add foo to apc!");
}
<?php
/*
* page2.php
* */
echo 'foo is been set as: ' . apc_fetch('foo');
p.s: i prefer to use apc_add
over apc_store
, but the only difference between them is that apc_add doesnt overwrite the variable but will fail if called twice with the same key:
Store the variable using this name. keys are cache-unique, so attempting to use apc_add() to store data with a key that already exists will not overwrite the existing data, and will instead return FALSE. (This is the only difference between apc_add() and apc_store().)
It's a matter of taste/task of the script, but the example above works with apc_store too.
Solution 2
You could always use an in-memory DB to save the data. Possibly overkill, though.
Solution 3
I am assuming you are on a shared server of some sort.
memcached
or another caching solution is indeed the only way to do this.
Sessions, the most prominent method of persisting data across PHP pages, work based on files. You can change the session handler to be database based instead, but that's not RAM based, either.
As far as I can see, without changing your system on root level (e.g. to install memcached, or store session files on a RAM disk), this is not possible.
Solution 4
Create a file in /dev/shm
and it will be kept in memory until the machine is rebooted. This may or may not be faster than using any old file, depending on your usage pattern.
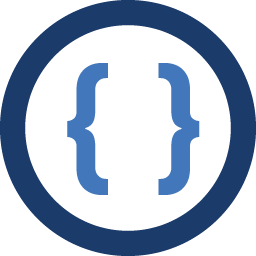
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
Is there a way to store small data in RAM memory using PHP so that I can have access to the data between different session instead of regenerating it. Something similar to memcached (I don't have access to memcahced). My current solution is just to save the data in file.