How can i toggle between any css property on click using javascript or jquery?
Solution 1
You may try something like this
HTML:
<button class="btn">Toggle Background</button>
CSS:
.btn{
background:#fff;
}
.btn_red{
background:red;
}
JS:
$(function(){
$('.btn').on('click', function(){
$(this).toggleClass('btn_red')
});
});
Vanilla JavaScript :
window.onload = function(){
document.querySelector('.btn').onclick = function(){
if(this.className.match('btn_red')) {
this.className = 'btn';
}
else {
this.className = 'btn btn_red';
}
};
};
DEMO. There are other ways but these are just some ideas.
Solution 2
A jQuery solution would be
http://jsfiddle.net/zMrtL/ (I put the text color to red so that on toggle it won't end up having text black on black i.e. invisible.)
CSS
#div { /* use any selector that matches your desired element */
background-color: #FFF;
}
#div.dark { /* the "dark" class to be added by jQuery,
put your above selector in front to increase specificity */
background-color: #000;
}
About the "specificity" issue, if you don't add #div
in front of dark
, the #div
definition will override the .dark
definition and the div will remain white, even it is added by jQuery. Look at this or this if you want to know more.
JavaScript/Jquery
// ensure HTML is ready/completely loaded, so that you won't end up
// binding click to something non-existent (at the time the binding occurs)
// Trying to bind something non-existent will not work because jQuery can never know
// if there is such element in the future, unless you use delegates (far fetched)
$(function(){
// on click, bind the "click" event to your element, and do something
$('#div').click(function(){
$(this).toggleClass('dark'); // on click, toggle the class of the element clicked
// i.e. if the class does not exist, add it, otherwise remove it.
// in jQuery, "this" is the element clicked/mouse-overed/etc.. i.e. target of the event
// in functions passed to jQuery event-binding functions.
// CSS/browsers is smart enough to detect these class changes and apply styles to new elements.
});
});
#div
means an element with id div
i.e. <div id="div"></div>
, as #
means an element that has the following ID. If you have class instead i.e. <div class="div"></div>
, use .div
Documentations for click
, toggleClass
, and passing a function to jQuery constructor
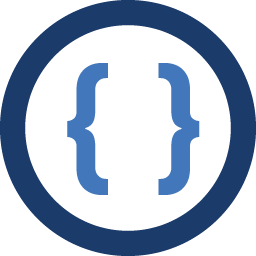
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
i am very new to javascript but i need something like suppose i have a white background button when i click it once then its background should change to black and when i click again on it its background should change into white again. means i need to change background of the same button on clicking the same button, please help me, i am really very puzzle and i am also in learning stage of javascript so if you can tell me something more about javascript or any idea beside what i need, i would be thankful to you, thanks.