How can I use pointers in Java?
Solution 1
All objects in Java are references and you can use them like pointers.
abstract class Animal
{...
}
class Lion extends Animal
{...
}
class Tiger extends Animal
{
public Tiger() {...}
public void growl(){...}
}
Tiger first = null;
Tiger second = new Tiger();
Tiger third;
Dereferencing a null:
first.growl(); // ERROR, first is null.
third.growl(); // ERROR, third has not been initialized.
Aliasing Problem:
third = new Tiger();
first = third;
Losing Cells:
second = third; // Possible ERROR. The old value of second is lost.
You can make this safe by first assuring that there is no further need of the old value of second or assigning another pointer the value of second.
first = second;
second = third; //OK
Note that giving second a value in other ways (NULL, new...) is just as much a potential error and may result in losing the object that it points to.
The Java system will throw an exception (OutOfMemoryError
) when you call new and the allocator cannot allocate the requested cell. This is very rare and usually results from run-away recursion.
Note that, from a language point of view, abandoning objects to the garbage collector are not errors at all. It is just something that the programmer needs to be aware of. The same variable can point to different objects at different times and old values will be reclaimed when no pointer references them. But if the logic of the program requires maintaining at least one reference to the object, It will cause an error.
Novices often make the following error.
Tiger tony = new Tiger();
tony = third; // Error, the new object allocated above is reclaimed.
What you probably meant to say was:
Tiger tony = null;
tony = third; // OK.
Improper Casting:
Lion leo = new Lion();
Tiger tony = (Tiger)leo; // Always illegal and caught by compiler.
Animal whatever = new Lion(); // Legal.
Tiger tony = (Tiger)whatever; // Illegal, just as in previous example.
Lion leo = (Lion)whatever; // Legal, object whatever really is a Lion.
Pointers in C:
void main() {
int* x; // Allocate the pointers x and y
int* y; // (but not the pointees)
x = malloc(sizeof(int)); // Allocate an int pointee,
// and set x to point to it
*x = 42; // Dereference x to store 42 in its pointee
*y = 13; // CRASH -- y does not have a pointee yet
y = x; // Pointer assignment sets y to point to x's pointee
*y = 13; // Dereference y to store 13 in its (shared) pointee
}
Pointers in Java:
class IntObj {
public int value;
}
public class Binky() {
public static void main(String[] args) {
IntObj x; // Allocate the pointers x and y
IntObj y; // (but not the IntObj pointees)
x = new IntObj(); // Allocate an IntObj pointee
// and set x to point to it
x.value = 42; // Dereference x to store 42 in its pointee
y.value = 13; // CRASH -- y does not have a pointee yet
y = x; // Pointer assignment sets y to point to x's pointee
y.value = 13; // Deference y to store 13 in its (shared) pointee
}
}
UPDATE: as suggested in the comments one must note that C has pointer arithmetic. However, we do not have that in Java.
Solution 2
As Java has no pointer data types, it is impossible to use pointers in Java. Even the few experts will not be able to use pointers in java.
See also the last point in: The Java Language Environment
Solution 3
Java does have pointers. Any time you create an object in Java, you're actually creating a pointer to the object; this pointer could then be set to a different object or to null
, and the original object will still exist (pending garbage collection).
What you can't do in Java is pointer arithmetic. You can't dereference a specific memory address or increment a pointer.
If you really want to get low-level, the only way to do it is with the Java Native Interface; and even then, the low-level part has to be done in C or C++.
Solution 4
There are pointers in Java, but you cannot manipulate them the way that you can in C++ or C. When you pass an object, you are passing a pointer to that object, but not in the same sense as in C++. That object cannot be dereferenced. If you set its values using its native accessors, it will change because Java knows its memory location through the pointer. But the pointer is immutable. When you attempt to set the pointer to a new location, you instead end up with a new local object with the same name as the other. The original object is unchanged. Here is a brief program to demonstrate the difference.
import java.util.*;
import java.lang.*;
import java.io.*;
class Ideone {
public static void main(String[] args) throws java.lang.Exception {
System.out.println("Expected # = 0 1 2 2 1");
Cat c = new Cat();
c.setClaws(0);
System.out.println("Initial value is " + c.getClaws());
// prints 0 obviously
clawsAreOne(c);
System.out.println("Accessor changes value to " + c.getClaws());
// prints 1 because the value 'referenced' by the 'pointer' is changed using an accessor.
makeNewCat(c);
System.out.println("Final value is " + c.getClaws());
// prints 1 because the pointer is not changed to 'kitten'; that would be a reference pass.
}
public static void clawsAreOne(Cat kitty) {
kitty.setClaws(1);
}
public static void makeNewCat(Cat kitty) {
Cat kitten = new Cat();
kitten.setClaws(2);
kitty = kitten;
System.out.println("Value in makeNewCat scope of kitten " + kitten.getClaws());
//Prints 2. the value pointed to by 'kitten' is 2
System.out.println("Value in makeNewcat scope of kitty " + kitty.getClaws());
//Prints 2. The local copy is being used within the scope of this method.
}
}
class Cat {
private int claws;
public void setClaws(int i) {
claws = i;
}
public int getClaws() {
return claws;
}
}
This can be run at Ideone.com.
Solution 5
Java does not have pointers like C has, but it does allow you to create new objects on the heap which are "referenced" by variables. The lack of pointers is to stop Java programs from referencing memory locations illegally, and also enables Garbage Collection to be automatically carried out by the Java Virtual Machine.
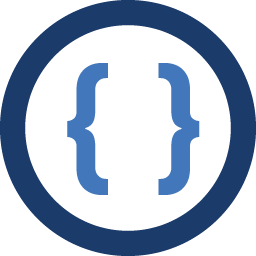
Admin
Updated on October 26, 2020Comments
-
Admin over 3 years
I know Java doesn't have pointers, but I heard that Java programs can be created with pointers and that this can be done by the few who are experts in java. Is it true?
-
David Seiler over 14 yearsNot even through JNI? No Java knowledge to speak of here, but I thought I'd heard you could get some low-level bit-grubbing done that way.
-
Poindexter over 14 yearsAs far as my ~4 years of Java experience and looking for the answer myself says no, Java pointers are not externally accessible.
-
Admin over 14 yearsI am curious to know that. Thanks for the answer
-
Admin over 14 yearsThanks for response. I asked because, my lecturer told that at research level where java used in handheld devices,,they use pointer...that;s why I asked
-
San Jacinto over 14 years@unknown(google) if it's at the research level, depending upon what is being done, it may have been that they needed such functionality, so they violated the normal idioms and implemented them anyway. You can implement your own JVM if you so wish to have a superset (or subset, or mixture) of normal Java features, but such code may not run on other Java platforms.
-
Admin over 14 years@san: Thanks for the answer. BTW I cannot vote..says that I need 15 reputation
-
R. Martinho Fernandes over 14 yearsNice answer, but you failed to address one key difference: C has pointer arithmetics. (Fortunately) you can't do that in Java.
-
Ethan Reesor about 12 yearsPointers are extremely powerful and useful. There are many cases where not having pointers (Java) makes code much less efficient. Just because people suck at using pointers doesn't mean languages should exclude them. Should I not have a rifle because others (like the military) use them to kill people?
-
Vishy about 12 yearsThere isn't much useful or powerful you can't do in Java as the language is. For everything else there is the Unsafe class which I find I need to use very rarely.
-
Danger almost 10 yearsI hate to down vote any answer, especially one that is popular, but Java does not have pointers and you simply can not use a reference like a pointer. There are certain things you can only do with real pointers - for example, you can get or set any byte in your process data space. You simply can't do that in Java. It's really bad that so many people here seem to not understand what a pointer really is, imho, and now I will get off my rant soap box and hope you forgive me for my little outburst.
-
user3462295 almost 10 yearsI think you mean Unfortunately. Why would you think it is good that java does not support pointer arithmetic?
-
iCodeSometime over 9 years@user3462295 Because people seem to think it is too difficult to be used by the general populace, and can only be understood by coding ninjas writing compilers or device drivers.
-
csga5000 almost 9 years@Danger He says "You can use these like a pointer", while it's true that it's only a reference, the general behavior of the object is the same, and it addresses the question properly.
-
Danger almost 9 years@csga5000 You misquoted the question. Nowhere in the question does the OP use the word "like". Therefore I believe both this answer and your comment are misleading and I will stand by my comment, which is: Java simply has no pointers. You can use a reference in some ways that are similar to pointers, but no, Java has no pointers which is what this question asked.
-
csga5000 almost 9 years@Danger First line of answer "All objects in Java are references and you can use them like pointers.". This is the best answer that can be provided. If the only answer had been "No, java has no pointers." that answer would have been unhelpful. This answer instead states what you can do instead.
-
csga5000 almost 9 years@Danger I did 1 up your comment when I read it, as I think it's a good point that java doesn't have pointers, that may have been better represented in the answer, however, the answer does not state that there ARE pointers, only that objects are references, and other helpful information.
-
Danger almost 9 yearsIt is true you can use references like pointers if you severely restrict how you use pointers, but then you're missing the point. (pun intended!) Thank you for 1-upping my comment though. A better and more clear answer would start with: "No, Java does not have pointers. But if you consider a restricted use of pointers which we can call references, than you can used a restricted subset of pointer functionality within Java."
-
Danger almost 9 yearsJava simply does not have pointers, plain and simple, and I can not for the life of me understand why so many answers here state incorrect information. This is StackOverlfow people! The definition of a pointer in computer science is (you can Google for this): "In computer science, a pointer is a programming language object, whose value refers to (or "points to") another value stored elsewhere in the computer memory using its address." A reference in Java is NOT a pointer. Java needs to run garbage collection and if you had a real pointer it would then be wrong!
-
Danger almost 9 yearsTechnically, no Java objects are pointers. They are references. If they were pointers, and your garbage collection ran, your program would instantly crash.
-
Danger almost 9 yearsNo, Java objects are not pointers. They are equivalent to references, which have a different set of capabilities and semantics.
-
Danger almost 9 yearsJava does need pointers, and that is why Java added JNI - to get around the lack of "pointer-like" or lower level functions you simply can not do in Java, no matter what code you write.
-
Danger almost 9 yearsOne of the few correct answers here hardly has any upvotes?
-
Danger almost 9 yearsMuch of the code I have written over the last 40 years could never have been implemented in Java, because Java is lacking core, low level capabilities.
-
Vishy almost 9 years@Danger which is why a large number of libraries use Unsafe and the idea of removing it has sparked so much debate in Java 9. The plan is to provide replacements, but they are not ready yet.
-
kingfrito_5005 almost 9 years@Danger A pointer is a a piece of data containing a memory address. Java is two things, which people forget. It is a language and a platform. Much as one would not say that .NET is a language, we need to remember that saying 'Java doesn't have pointers' can be misleading because the platform does of course have and use pointers. Those pointers are not accessible to the programmer through the Java language, but they do exist in the runtime. References in the language exist on top of actual pointers in the runtime.
-
kingfrito_5005 almost 9 yearsNote that there exist 'pointer' objects that simulate pointerlike behavior. For many purposes this can be used the same way, memory level addressing not withstanding. Given the nature of the question I feel its odd that nobody else mentioned this.
-
Danger almost 9 years@kingfrito_5005 It would appear that you are agreeing with me. I believe you said "Those pointers are not accessible to the programmer through the Java Language" which is consistent with my statement that "Java does not have pointers". References are semantically significantly different from pointers.
-
kingfrito_5005 almost 9 years@Danger this is true, but I think its important in these cases to distinguish between the platform and the language because I know that when I was first starting out a statement like yours would have confused me.
-
Neeraj Yadav over 6 yearsThis should be the answer at top. Because for beginners telling that "behind the scenes java have pointers" can lead to very confusing state. Even when Java is taught at starting level, it is taught that pointers are not secure that's why they are not used in Java. A programmer needs to be told what he/she can see and work on. Reference and pointers are actually very different.
-
Sometowngeek almost 5 yearsNot sure I entirely agree with the "2.2.3 No Enums" topic in the link. The data might be outdated, but Java does support enums.
-
Fortega almost 5 years@Sometowngeek the document linked to is a white paper from 1996 describing the very first version of java. Enums are introduced in java version 1.5 (2004)
-
Sometowngeek almost 5 years@Fortega derp... I didn't realize the document was dated. Thanks for clarifying :)
-
VulstaR over 4 yearsYup, Java certainly has pointers behind the variables. It is just that developers dont have to deal with them because java is doing the magic for this behind the scenes. This means developers are not directly allowed to point a variable to a specific memmory adress, and deal with data offsets and stuff. It allows java to keep a clean memmory garbage collection, but since every variable is essentialy a pointer, it also takes some extra memmory to have all those auto generated pointer adresses, which in the more low level languages could have been avoided.
-
italianfoot about 4 yearsCould you elaborate on the aliasing problem?
-
Michael Tsang about 4 yearsJava has pointers.
Dog d = new Dog(); d.bark();
in Java is the same asDog *d = new Dog(); d->bark();
in C++. The difference is that, in Java you can't take a reference to get a pointer to non-objects, and you can't deference object pointers. Also you can't convert to numbers and do maths on it. Accessing an object thru anull
pointer will get aNullPointerException
. -
Michael Tsang about 4 yearsThis is plain wrong. You can't write a
swap
function in Java which will swap two objects. -
PeMa over 3 yearsThis actually is the only correct answer. I was just about to write an answer pointing to baeldung.com/java-unsafe.
-
Vishy over 3 years@PeMa about 5 years ago I wrote a library to wrap much of this functionality to make it safer. github.com/OpenHFT/Chronicle-Bytes
-
PeMa over 3 years@PeterLawrey thank you for the comment. I'll have a closer look at it.
-
Vishy over 3 years@PeMa I suggest you also look at Chronicle Queue and Chronicle Map which use this library to store data in off heap and persisted shared memory.
-
niken about 3 yearsStop marketing your software and just admit pointers in java would change the game. And , dare I say , make (most) of the library you have worked so hard on obsolete ? Don't worry though, "they" won't add pointers any time soon, because come on, just read some of the answers and comments ( lol... )
-
Vishy about 3 years@niken Mentioning software in a comment 11 years after the original answer isn't marketing.
-
Vishy about 3 years@niken In May it had 80K downloads, not too obsolete.
-
ttbek over 2 yearsI might be a weird case, but I've had more memory leaks using Java than C or C++ because the garbage collector wasn't triggering. I seem to always end up need to explicitly call the garbage collector. Not that I haven't had other pointer related errors, but specifically for leaks I've had them more often with Java than without.
-
Robherc KV5ROB about 2 yearsI think the answer should be updated from "This is very rare and usually only results from run away recursion" to "This is very rare and usually only results from infinite loops or run away recursion" lol