How can you adjust Android SnackBar to a specific position on screen
Solution 1
It is possible to set the location that the Snackbar is displayed by positioning a android.support.design.widget.CoordinatorLayout
within your existing Activity layout.
For example, say your existing layout is a RelativeLayout
you could add a CoordinatorLayout
as follows:
<android.support.design.widget.CoordinatorLayout
android:layout_width="match_parent"
android:layout_height="200dp"
android:id="@+id/myCoordinatorLayout"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true">
</android.support.design.widget.CoordinatorLayout>
Then, make sure you pass the CoordinatorLayout
as the first argument of the Snackbar.make()
command.
final View viewPos = findViewById(R.id.myCoordinatorLayout);
Snackbar.make(viewPos, R.string.snackbar_text, Snackbar.LENGTH_LONG)
.setAction(R.string.snackbar_action_undo, showListener)
.show();
This will result in the Snackbar being shown at the bottom of the CoordinatorLayout
provided to the make() function.
If you pass a View
that is not a CoordinatorLayout
the Snackbar will walk up the tree until it finds a CoordinatorLayout
or the root of the layout.
Solution 2
Defining a layout explicitly for the Snackbar may not always be practical.
The issue seems to have been targeting the parent, rather than the Snackbar.
Snackbar snackbar = Snackbar.make(layout, message, duration);
View snackbarLayout = snackbar.getView();
LinearLayout.LayoutParams lp = new LinearLayout.LayoutParams(
LinearLayout.LayoutParams.WRAP_CONTENT,
LinearLayout.LayoutParams.WRAP_CONTENT
);
// Layout must match parent layout type
lp.setMargins(50, 0, 0, 0);
// Margins relative to the parent view.
// This would be 50 from the top left.
snackbarLayout.setLayoutParams(lp);
snackbar.show();
Solution 3
I have an interesting idea for you.
You can just use the code below to get where you want.
Snackbar.make(View,Message , Snackbar.LENGTH_LONG)
.setAnchorView(pb)
.show();
Here setAnchorView
is used to change the postion. You can create your layout in the xml file. Just call your layout inside the setAnchorView
and it will show on the position of the xml layout.
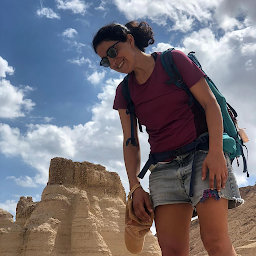
Dus
Updated on November 10, 2021Comments
-
Dus over 2 years
Follwing the new android snackbar, i'm trying to set it to be positioned on a specific y coordinate. Its seems to be not even a possible.
I've tried with getting the parent of the snackbar's view, but, there's nothing to be done to the parent for it to set the position of it.
mSnackBar.getView().getParent();
When digging into the actual class, there's an instance of mView, and mParent which is private, and can't be reached anyhow.
https://developer.android.com/reference/android/support/design/widget/Snackbar.html