How can you save out a String into a file in AS3?
Solution 1
Yes you can, with FileReference. This is basically how it's done:
var bytes:ByteArray = new ByteArray();
var fileRef:FileReference=new FileReference();
fileRef.save("fileContent", "fileName");
Doesn't look too hard, does it? And here's a video-tutorial on it too:
http://www.gotoandlearn.com/play?id=76
And the documentation:
http://livedocs.adobe.com/flash/9.0/ActionScriptLangRefV3/
Hope that helps.
Solution 2
Since I had a function to output bytes to a file (because I was doing something with bitmaps), I reused it to output a string as well, like this:
var filename:String = "/Users/me/path/to/file.txt"; var byteArray:ByteArray = new ByteArray(); byteArray.writeUTFBytes(someString); outFile(filename, byteArray); private static function outFile(fileName:String, data:ByteArray):void { var outFile:File = File.desktopDirectory; // dest folder is desktop outFile = outFile.resolvePath(fileName); // name of file to write var outStream:FileStream = new FileStream(); // open output file stream in WRITE mode outStream.open(outFile, FileMode.WRITE); // write out the file outStream.writeBytes(data, 0, data.length); // close it outStream.close(); }
Solution 3
In addition, you must have Flash Player 10 and a Flex Gumbo SDK installed in your Flex Builder 3.
You can also have a look the following example: http://blog.flexexamples.com/2008/08/25/saving-files-locally-using-the-filereference-classs-save-method-in-flash-player-10/
Solution 4
In Flex 3 no you can't do it unless you upload the file to the server and then download the file via a url to the desktop.
In Air or Flex 4 you can save it directly from the application to the desktop as detailed above.
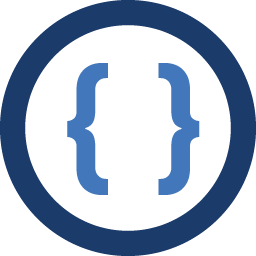
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
I have a string the user has typed and I want to save it into a file on the users harddrive. Can you do that? And if so, how?
-
Yozef over 10 yearsthe link provided is a dead link
-
AlphaCactus over 5 yearsWhat is the purpose of
var bytes
in the example?