How cancel Downloading Async?
Solution 1
When you call CancelAsync
, the AsyncCompletedEventArgs
object passed to the completed callback will have the Cancelled
property set to true. So you could write:
void client_InstallFileCompleted(object sender, AsyncCompletedEventArgs e)
{
if(e.Cancelled)
{
// delete the partially-downloaded file
return;
}
// unzip and do whatever...
using (ZipFile zip = ZipFile.Read(@"C:\U.Rage\Downloads\" + installID + "Install.zip"))
See the documentation for more info.
Solution 2
Here is the method for async data download that supports cancellation:
private static async Task<byte[]> downloadDataAsync(Uri uri, CancellationToken cancellationToken)
{
if (String.IsNullOrWhiteSpace(uri.ToString()))
throw new ArgumentNullException(nameof(uri), "Uri can not be null or empty.");
if (!Uri.IsWellFormedUriString(uri.ToString(), UriKind.Absolute))
return null;
byte[] dataArr = null;
try
{
using (var webClient = new WebClient())
using (var registration = cancellationToken.Register(() => webClient.CancelAsync()))
{
dataArr = await webClient.DownloadDataTaskAsync(uri);
}
}
catch (WebException ex) when (ex.Status == WebExceptionStatus.RequestCanceled)
{
// ignore this exception
}
return dataArr;
}
Solution 3
The selected answer didn't work properly for me. Here's what I did:
When they click the cancel button I call the
Client.CancelAsync();
And then in the Web.Client DownloadFileCompleted:
Client.DownloadFileCompleted += (s, e) =>
{
if (e.Cancelled)
{
//cleanup delete partial file
Client.Dispose();
return;
}
}
And then when you try to re-download just instantiate a new client:
Client = WebClient();
This way the old async parameters won't be maintained.
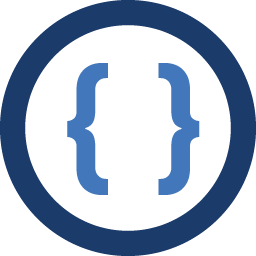
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
I've got a problem. How can I cancel a download ?
client.CancelAsync();
Doesn't work for me, because if I cancel a download and start a new one the code still tries to access the old download file. You've to know in my code is a part when a download is done it should unzip the file which has been downloaded. Example.zip like this :) So, when I cancel my download and start a new one you know my script tries to unzip my old Example.zip, but it should kick this ....
For Unzipping, I'm using Iconic.Zip.dll (http://dotnetzip.codeplex.com/)
How to get it work?
UPDATE:
This is my Downloader Form
private void button3_Click_1(object sender, EventArgs e) { DialogResult dialogResult = MessageBox.Show("This will cancel your current download ! Continue ?", "Warning !", MessageBoxButtons.YesNo); if (dialogResult == DialogResult.Yes) { cancelDownload = true; URageMainWindow.isDownloading = false; this.Close(); } else if (dialogResult == DialogResult.No) { } }
This is my Main form this happens when you start downloading something
private void checkInstall(object sender, WebBrowserDocumentCompletedEventArgs e) { string input = storeBrowser.Url.ToString(); // Test these endings string[] arr = new string[] {"_install.html"}; // Loop through and test each string foreach (string s in arr) { if (input.EndsWith(s) && isDownloading == false) { // MessageBox.Show("U.Rage is downloading your game"); Assembly asm = Assembly.GetCallingAssembly(); installID = storeBrowser.Document.GetElementById("installid").GetAttribute("value"); // MessageBox.Show("Name: " + installname + " ID " + installID); installname = storeBrowser.Document.GetElementById("name").GetAttribute("value"); installurl = storeBrowser.Document.GetElementById("link").GetAttribute("value"); isDownloading = true; string install_ID = installID; string Install_Name = installname; // MessageBox.Show("New Update available ! " + " - Latest version: " + updateversion + " - Your version: " + gameVersion); string url = installurl; WebClient client = new WebClient(); client.DownloadProgressChanged += new DownloadProgressChangedEventHandler(client_InstallProgressChanged); client.DownloadFileCompleted += new AsyncCompletedEventHandler(client_InstallFileCompleted); client.DownloadFileAsync(new Uri(url), @"C:\U.Rage\Downloads\" + installID + "Install.zip"); if (Downloader.cancelDownload == true) { //MessageBox.Show("Downloader has been cancelled"); client.CancelAsync(); Downloader.cancelDownload = false; } notifyIcon1.Visible = true; notifyIcon1.ShowBalloonTip(2, "Downloading !", "U.Rage is downloading " + installname, ToolTipIcon.Info); System.Media.SoundPlayer player = new System.Media.SoundPlayer(@"c:/U.Rage/Sounds/notify.wav"); player.Play(); storeBrowser.GoBack(); igm = new Downloader(); igm.labelDWGame.Text = installname; // this.Hide(); igm.Show(); return; } if (input.EndsWith(s) && isDownloading == true) { System.Media.SoundPlayer player = new System.Media.SoundPlayer(@"c:/U.Rage/Sounds/notify.wav"); player.Play(); MessageBox.Show("Please wait until your download has been finished", "Warning"); storeBrowser.GoBack(); } } }
This happens when the download has been finished
void client_InstallFileCompleted(object sender, AsyncCompletedEventArgs e) { if(Downloader.cancelDownload == false) { using (ZipFile zip = ZipFile.Read(@"C:\U.Rage\Downloads\" + installID + "Install.zip")) { //zip.Password = "iliketrains123"; zip.ExtractAll("C:/U.Rage/Games/", ExtractExistingFileAction.OverwriteSilently); } System.IO.File.Delete(@"C:/U.Rage/Downloads/" + installID + "Install.zip"); notifyIcon1.Visible = true; notifyIcon1.ShowBalloonTip(2, "Download Completed !", "Installing was succesful !", ToolTipIcon.Info); System.Media.SoundPlayer player = new System.Media.SoundPlayer(@"c:/U.Rage/Sounds/notify.wav"); player.Play(); this.Show(); igm.Close(); isDownloading = false; listView.Items.Clear(); var files = Directory.GetFiles(@"C:\U.Rage\Games\", "*.ugi").Select(f => new ListViewItem(f)).ToArray(); foreach (ListViewItem f in files) { this.LoadDataFromXml(f); } } }