How do Arrays work in the "for" loop (C language)
Solution 1
You should use
int input[2];
since you want an array of two integers. Otherwise , it will just allocate one integer array for you, and accessing input[1] in that case will not work properly.
Also try this to extend that to adding n integers.
int main()
{
int input[1];
int total = 0;
int n; //To count number of elements to add:
printf("Please enter the number of elements to add: ");
scanf("%d", &n);
for(int i = 0; i < n; i++)
{
printf("Please enter integer %d: ",(i+1));
scanf("%d", &input[i]);
}
for(int i = 0; i < n; i++)
{
total = total +input[i];
}
printf("The sum is = ", total);
}
So in your array, as indicated in the loops I have used, you will be accessing a single element of input array in each iteration by making use of its index which you also increment in each iteration.
In your case, there are only two elements, so it requires two iterations only.
Solution 2
this line
int input[1];
will create array of size one only. And i can see you are trying to access second element of array also.
so just replace your this line with
int input[2];
this will solve your problem. or put this whole thing
int main()
{
int input[2];
for(int i = 0; i < 2; i++)
{
printf("Please enter an integer: ");
scanf("%d", &input[i]);
}
int total = input[0]+input[1];
printf("%d + %d = %d ", input[0], input[1], total);
}
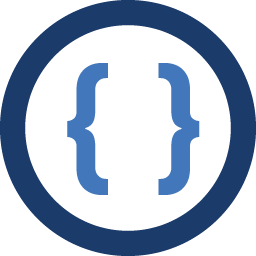
Admin
Updated on January 20, 2020Comments
-
Admin over 4 years
i am relatively new in c programming, and programming in general.
1) I am trying to create an array named "input" that will be used for the user input (and the array will only use two integer elements).
2) I want to use the for loop so it loop through my code 2 times, so i can duplicate the printf statement "Enter an integer," without me typing the printf statement multiple times. And then my scanf placeholder will be based my array "input."
3) Then i want to add those two numbers together for the sum.
The problem: When the user enters a number, for example, 1 and then 1 again, the index at input[0] is 1 but then the index at 1 for input[1] is 2...
I will use addition to try and explain. When i try to output the numbers in addition (integer[0] is 1, integer[1] is 2 and my variable which adds them together is "total")i get this: 1+2=2.
So why is input[0] correct, but input[1] seems to be adding the two user inputs together and storing it in there?
Here is my code
int main() { int input[1]; for(int i = 0; i < 2; i++) { printf("Please enter an integer: "); scanf("%d", &input[i]); } int total = input[0]+input[1]; printf("%d + %d = %d ", input[0], input[1], total); }
Thanks in advance, and i hope you understand me. Sorry i'm a noob, learning the basics so i can become advanced.