How do I add time-stamp information to Maven artifacts?
Solution 1
Maven versions 2.1.0-M1 or newer have built in special variable maven.build.timestamp
.
<build>
<finalName>${project.artifactId}-${project.version}-${maven.build.timestamp}</finalName>
</build>
See Maven documentation for more details.
For older Maven versions a look at maven-timestamp-plugin or buildnumber-maven-plugin.
If you use maven-timestamp-plugin, you can use something like this to manipulate resulting artifact name.
<build>
<finalName>${project.artifactId}-${project.version}-${timestamp}</finalName>
</build>
And this configuration for buildnumber-maven-plugin should create a ${timestamp} property which contains the timestamp value. There doesn't seem to be a way to create the version.properties file directly with this plugin.
<configuration>
<format>{0,date,yyyyMMddHHmmss}</format>
<items>
<item>timestamp</item>
</items>
</configuration>
These three sites are also worth checking out.
Solution 2
If you use a version of Maven >= 2.1.0-M1, then you can use the ${maven.build.timestamp} property.
For more info, see: http://maven.apache.org/guides/introduction/introduction-to-the-pom.html#Available_Variables
Solution 3
This post (especially the below part) is also very useful and practical for this issue.
Stamping Version Number and Build Time in a Properties File with Maven
The pom will look like this
...
<properties>
....
<!-- Timestamp of build -->
<timestamp>${maven.build.timestamp}</timestamp>
<maven.build.timestamp.format>yyyy_MM_dd_HH_mm</maven.build.timestamp.format>
</properties>
...
<build>
<finalName>${project.artifactId}-${project.version}-${timestamp}</finalName>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
....
</plugin>
</plugins>
</build>
....
and the package name is MyProject-1.0. 0-2015_03_02_13_46.war
Solution 4
If you need the time in a timezone other than UTC (the default when you use ${maven.build.timestamp}
) you could use the build-helper-maven-plugin
. See more in Brief examples on how to use the Build Helper Maven Plugin's goals.
Anyway, this is how I've got the timestamp in GMT-5 and put it in the final name of my artifact:
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>build-helper-maven-plugin</artifactId>
<version>1.9.1</version>
<executions>
<execution>
<id>timestamp-property</id>
<goals>
<goal>timestamp-property</goal>
</goals>
<configuration>
<name>current.time</name>
<pattern>yyyyMMdd-HHmmss</pattern>
<timeZone>GMT-5</timeZone>
</configuration>
</execution>
</executions>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<version>2.4</version>
<configuration>
<finalName>${project.name}-${current.time}</finalName>
</configuration>
</plugin>
</plugins>
</build>
Solution 5
When a SNAPSHOT project is deployed, by default a timestamp is used unless you override it in the deploy plugin. If you're not getting unique timestamps, it is probably down to a configuration of your Maven repository. As the other answer says though, use the timestamp or buildnumber plugin for releases.
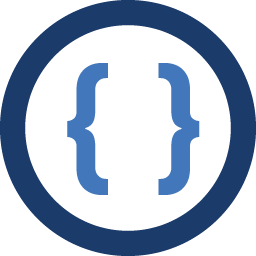
Admin
Updated on March 18, 2020Comments
-
Admin about 4 years
I am upgrading a large build-system to use Maven2 instead of Ant, and we have two related requirements that I'm stuck on:
We need to generate a time-stamped artifact, so a part of the package phase (or wherever), instead of building
project-1.0-SNAPSHOT.jar
we should be building
project-1.0-20090803125803.jar
(where the
20090803125803
is just aYYYYMMDDHHMMSS
time-stamp of when the jar is built).The only real requirement is that the time-stamp be a part of the generated file's filename.
The same time-stamp has to be included within a version.properties file inside the generated jar.
This information is included in the generated pom.properties when you run, e.g.,
mvn package
but is commented out:#Generated by Maven #Mon Aug 03 12:57:17 PDT 2009
Any ideas on where to start would be helpful! Thanks!
-
Mike Cornell over 14 yearsYou may be able to create your version.properties file by stubbing it out in /src/main/resources and using filtering. Then use the ${timestamp} property in the filter. The trick would be getting the ${timestamp} property created before the filters are applied.
-
Xorty almost 10 yearsI prefer this one as no plugin is needed.
-
fl4l over 9 yearsIf you use maven-war-plugin just add this in configuration:
<configuration>...<warName>${project.name}-${project.version}_${maven.build.timestamp}</warName></configuration>
-
lmiguelmh over 9 yearsThis will always result the time in UTC format, if you want the time in another timezone use the
build-helper-maven-plugin
plugin: Search for timezone in http://mojo.codehaus.org/build-helper-maven-plugin/usage.html. -
Jan almost 8 yearsJust in case some stumbles over this old answer like me: It is now build in: maven.apache.org/guides/introduction/…
-
gmanjon almost 7 yearsBut wouldn't
${project.artifactId}-${project.version}-${timestamp}
will generateproject-1.0-SNAPSHOT-20090803125803
? -
Gary Sheppard almost 6 years@lmiguelmh "This will always result the time in UTC format" I use Maven 3.0.5 and
${maven.build.timestamp}
displays in the local time zone, not UTC. Maybe that's a recent change in Maven. -
Ed Randall about 5 yearsThe format of the build timestamp can be customized by declaring the property
maven.build.timestamp.format
which follows JavaSimpleDateFormat
patterns -
ChRoNoN almost 5 yearsNo clue why, but Maven's timestamp does not use current timezone, so I would not consider this answer a solution
-
not2savvy over 4 yearsDoesn't work like this here. Can you elaborate your answer?
-
user1062589 over 3 yearsLink to post is broken. Link that works: dzone.com/articles/stamping-version-number-and