How do I call a non static method from a main method?
39,580
Solution 1
You can't. A non-static method is one that must be called on an instance of your Test class; create an instance of Test to play with in your main method:
public class Test {
public static void main(String args[]) {
int[] arr = new int[5];
arr = new int[] { 1, 2, 3, 4, 5 };
Test test = new Test();
test.arrPrint(arr);
}
public void arrPrint(int[] arr) {
for (int i = 0; i < arr.length; i++)
System.out.println(arr[i]);
}
}
Solution 2
You can call non-static method only using a class instance, so you have to create it using new keyword.
public class Something {
public static void main(String args[]) {
Something something = new Something();
something.method1();
new Something().method2();
}
public void method1() {
}
public void method2() {
}
}
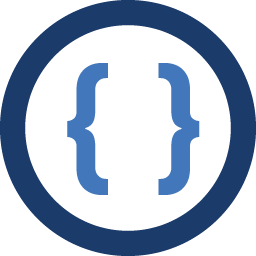
Author by
Admin
Updated on April 09, 2020Comments
-
Admin about 4 years
For example, I am trying to do something like this
public class Test { public static void main(String args[]) { int[] arr = new int[5]; arrPrint(arr); } public void arrPrint(int[] arr) { for (int i = 0; i < arr.length; i++) System.out.println(arr[i]); } }
I get an error telling me that I can't reference non-static variables from static enviorments. So if that is true how would I ever utilize a non static method inside of a main?