How do I call this IEnumerator in unity c#
Solution 1
It looks like you aren't keeping your Coroutine alive after you have called it in Start. The call to StartCoroutine will execute once then return, so without a loop of some sort you will only have one call to the coroutine body.
Enclosing the yield statement in a loop will give you one iteration per the waitTime specified. In the Example below you can see that the console logs timestamp updates once every 3 seconds.
using UnityEngine;
using System.Collections;
public class NewBehaviourScript : MonoBehaviour {
// Use this for initialization
void Start () {
StartCoroutine(ResourceTickOver(3.0f));
}
// Update is called once per frame
void Update () {
}
IEnumerator ResourceTickOver(float waitTime)
{
while (true) // Do this as long this script is running.
{
print (Time.time);
yield return new WaitForSeconds(waitTime);
print (Time.time);
// Update Resources inside this loop or call something that will.
}
}
}
Solution 2
First way using coroutine:
void Start () {
StartCoroutine(ResourceTickOver(3.0f));
}
IEnumerator ResourceTickOver(float waitTime){
while(true){
yield return new WaitForSeconds(waitTime);
foodTotal += foodPtick;
PowerTotal += PowerPtick;
HappinessTotal += HappinessPtick;
MoneyTotal += MoneyPtick;
PopulationTotal += PopulationPtick;
Debug.Log("Resources" + "food" + foodTotal + "power" + PowerTotal + "Happiness" + HappinessTotal + "Money" + MoneyTotal + "Population" + PopulationTotal);
}
}
second way using Update:
void Update(){
timer += Time.deltaTime;
if(timer > waitTime){
timer = 0f;
foodTotal += foodPtick;
PowerTotal += PowerPtick;
HappinessTotal += HappinessPtick;
MoneyTotal += MoneyPtick;
PopulationTotal += PopulationPtick;
Debug.Log("Resources" + "food" + foodTotal + "power" + PowerTotal + "Happiness" + HappinessTotal + "Money" + MoneyTotal + "Population" + PopulationTotal);
}
}
third way with InvokeRepeating:
void Start(){
InvokeRepeating("Method", 3f, 3f);
}
void Method(){
foodTotal += foodPtick;
PowerTotal += PowerPtick;
HappinessTotal += HappinessPtick;
MoneyTotal += MoneyPtick;
PopulationTotal += PopulationPtick;
Debug.Log("Resources" + "food" + foodTotal + "power" + PowerTotal + "Happiness" + HappinessTotal + "Money" + MoneyTotal + "Population" + PopulationTotal);
}
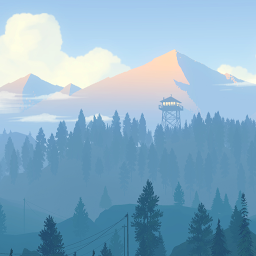
Andrew Sutcliffe
Updated on July 16, 2022Comments
-
Andrew Sutcliffe almost 2 years
So I am trying to Increment my total resources every 3 seconds by my PTick variables I tried using it via an IEnumerator and calling it inside the the start method but it only runs once so I tried it in the update and it runs as quickly as it can. is there any way I can get it just to run every 3 seconds. im happy to try alternatives so long as I can get it running every 3 seconds.
this is the script That I'm trying to get working
using UnityEngine; using System.Collections; public class Resources : MonoBehaviour { private int foodPtick; private int PowerPtick; private int HappinessPtick; private int MoneyPtick; private int PopulationPtick; public int foodTotal; public int PowerTotal; public int HappinessTotal; public int MoneyTotal; public int PopulationTotal; void Start () { StartCoroutine(ResourceTickOver(3.0f)); } void Update () { } IEnumerator ResourceTickOver(float waitTime){ yield return new WaitForSeconds(waitTime); foodTotal += foodPtick; PowerTotal += PowerPtick; HappinessTotal += HappinessPtick; MoneyTotal += MoneyPtick; PopulationTotal += PopulationPtick; Debug.Log("Resources" + "food" + foodTotal + "power" + PowerTotal + "Happiness" + HappinessTotal + "Money" + MoneyTotal + "Population" + PopulationTotal); } public void ChangeResource(int food,int power, int happy, int money,int pop) { Debug.Log("Old Per Tick" + "food" + foodPtick + "power" + PowerPtick + "Happiness" + HappinessPtick + "Money" + MoneyPtick + "Power" + PopulationPtick); foodPtick += food; PowerPtick += power; HappinessPtick += happy; MoneyPtick += money; PopulationPtick += pop; Debug.Log("New Per Tick" + "food" + foodPtick + "power" + PowerPtick + "Happiness" + HappinessPtick + "Money" + MoneyPtick + "Power" + PopulationPtick); }