How do I check if a Kendo Grid has had changes made to it?
Solution 1
Added rows will have the dirty property set to true and so will updated rows. But, deleted rows are stored elsewhere (in the _destroyed collection). Pass this function the datasource of your grid to see if it has changes.
function doesDataSourceHaveChanges(ds)
{
var dirty = false;
$.each(ds._data, function ()
{
if (this.dirty == true)
{
dirty = true;
}
});
if (ds._destroyed.length > 0) dirty = true;
return dirty;
}
Solution 2
You can use the 'hasChanges' method on the Grid's underlying DataSource:
grid.dataSource.hasChanges();
$('#divGrid').data('kendoGrid').dataSource.hasChanges();
Solution 3
You can get notified and use the change event of the dataSource which will occur wherever you page/sort/group/filter/create/read/update/delete record.
To attach a handler to it use:
$('#YourGrid').data().kendoGrid.dataSource.bind('change',function(e){
//the event argument here will indicate what action just happned
console.log(e.action)// could be => "itemchange","add" or "remove" if you made any changes to the items
})
Update: If the user has updated any of the models .hasChanges() method of the dataSource will return true.
Solution 4
grid.dataSource.hasChanges will let you know if the datasource has changed
if (datasource.hasChanges() === true) {
alert('yes');
} else {
alert('no');
}
Solution 5
The most convinient way to go is to use datasource.hasChanges()
. However, this requires that the Id
field is defined in the schema.
From the docs:
Checks if the data items have changed. Requires an [ID field] to be configured in schema.model.id, otherwise will always return true.
If you do not have an Id field defined, you can use one of the countless ways to iterate over the data itself.
var isDataSourceDirty = function (ds) {
var dirty = ds._data.filter(function(x){
return x.dirty === true;
});
return dirty.length > 0 || ds._destroyed.length;
};
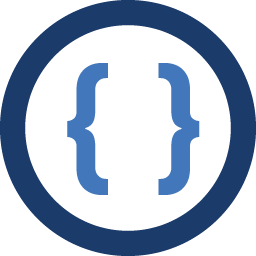
Admin
Updated on July 17, 2022Comments
-
Admin almost 2 years
How can I check if a Kendo Grid has changes? I heard that there is a
dirty
property, but I cant find it.