How do I convert a UITextField to an integer in Swift 2.0?
Solution 1
To convert the input string to an integer using Swift 2:
let guess:Int? = Int(input.text)
if guess == randomNumber {
// your code here
}
Solution 2
To access it's int value:
Int(input.text)
The input variable has type UITextField, which is an object and can't be compared to an Int. So you have to access its text, which is an string, and cannot be compared to Int as well, so, convert it to an Int using the expression above, it will give you the int value that the string holds.
Solution 3
You can convert string into int using this:
input.text.toInt()!
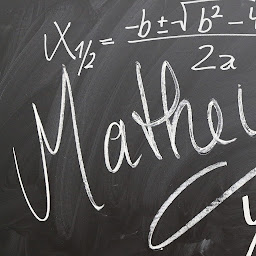
Joe Maglione
Updated on September 26, 2020Comments
-
Joe Maglione over 3 years
I'm a beginner at swift, and was doing some testing. To tell you the truth, I barely know what I'm doing yet, so please try to explain clearly. I was making a random number generator app & wanted to add in a
uitextfield
so that the user can type in their guess of the next randomly generated number. I keep getting an error when trying to use the if statement to compare the randomly generated number to the number entered In the text field.import UIKit class ViewController: UIViewController, UITextFieldDelegate { @IBOutlet weak var input: UITextField! @IBOutlet weak var infoLabel: UILabel! // Displayed Before The User Clicks Button For The First Time. @IBOutlet weak var numberLabel: UILabel! @IBAction func go(sender: AnyObject) { // Remove The Text Under The Button infoLabel.text = " " // Generate Random Number let randomNumber = Int(arc4random_uniform(11)) // Change The "Number Label's" Text In Order To Show The Randomly Generated Number To The User numberLabel.text = "\(randomNumber)" // Check To See If The User Guessed The Correct Number, And If They Did, Tell Them They Were Right if input == randomNumber { infoLabel.text = "Your Guess,\(randomNumber) Was Correct!" } } override func viewDidLoad() { super.viewDidLoad() } }
-
nick almost 9 yearsTo whoever downvoted this, can you explain why? I don't see anything wrong with his answer.
-
Mick MacCallum almost 9 years@nick Perhaps because the question is asking about Swift 2, in which
toInt()
has been removed. -
Joe Maglione almost 9 yearsThanks SO MUCH!!! I had to just put an exclamation point after input.text, but after that it worked perfectly.
-
Eric Aya over 7 yearsNo, not in Swift 2 - this is for Swift 1.