How do I create RAW TCP/IP packets in C++?
Solution 1
Start by reading Beej's guide on socket programming . It will bring you up to speed on how to start writing network code. After that you should be able to pick up more and more information from reading the man pages.
Most of it will consist of reading the documentation for your favourite library, and a basic understanding of man pages.
Solution 2
For TCP client side:
Use gethostbyname to lookup dns name to IP, it will return a hostent structure. Let's call this returned value host.
hostent *host = gethostbyname(HOSTNAME_CSTR);
Fill the socket address structure:
sockaddr_in sock;
sock.sin_family = AF_INET;
sock.sin_port = htons(REMOTE_PORT);
sock.sin_addr.s_addr = ((struct in_addr *)(host->h_addr))->s_addr;
Create a socket and call connect:
s = socket(AF_INET, SOCK_STREAM, 0);
connect(s, (struct sockaddr *)&sock, sizeof(sock))
For TCP server side:
Setup a socket
Bind your address to that socket using bind.
Start listening on that socket with listen
Call accept to get a connected client. <-- at this point you spawn a new thread to handle the connection while you make another call to accept to get the next connected client.
General communication:
Use send and recv to read and write between the client and server.
Source code example of BSD sockets:
You can find some good example code of this at wikipedia.
Further reading:
I highly recommend this book and this online tutorial:
4:
Solution 3
A good place to start would be to use Asio which is a great cross-platform (incl Linux) library for network communication.
Solution 4
I've been developing libtins for the past year. It's a high level C++ packet crafting and sniffing library.
Unless you want to reinvent the wheel and implement every protocol's internals, I'd recommend you to use some higher level library which already does that for you.
Solution 5
You do this the exact same way you would in regular C, there is no C++ specific way to do this in the standard library.
The tutorial I used for learning this was http://beej.us/guide/bgnet/. It was the best tutorial I found after looking around, it gave clear examples and good explanations of all functions it describes.
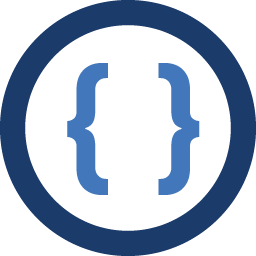
Admin
Updated on August 03, 2022Comments
-
Admin almost 2 years
I'm a beginning C++ programmer / network admin, but I figure I can learn how to do this if someone points me in the right direction. Most of the tutorials are demonstrated using old code that no longer works for some reason.
Since I'm on Linux, all I need is an explanation on how to write raw Berkeley sockets. Can someone give me a quick run down?