How do I divide so I get a decimal value?
Solution 1
quotient = 3 / 2;
remainder = 3 % 2;
// now you have them both
Solution 2
In your example, Java is performing integer arithmetic, rounding off the result of the division.
Based on your question, you would like to perform floating-point arithmetic. To do so, at least one of your terms must be specified as (or converted to) floating-point:
Specifying floating point:
3.0/2
3.0/2.0
3/2.0
Converting to floating point:
int a = 2;
int b = 3;
float q = ((float)a)/b;
or
double q = ((double)a)/b;
(See Java Traps: double and Java Floating-Point Number Intricacies for discussions on float
and double
)
Solution 3
Check this out: http://download.oracle.com/javase/1,5.0/docs/api/java/math/BigDecimal.html#divideAndRemainder%28java.math.BigDecimal%29
You just need to wrap your int or long variable in a BigDecimal object, then invoke the divideAndRemainder method on it. The returned array will contain the quotient and the remainder (in that order).
Solution 4
Don't worry about it. In your code, just do the separate / and % operations as you mention, even though it might seem like it's inefficient. Let the JIT compiler worry about combining these operations to get both quotient and remainder in a single machine instruction (as far as I recall, it generally does).
Solution 5
int a = 3;
int b = 2;
float c = ((float)a)/b
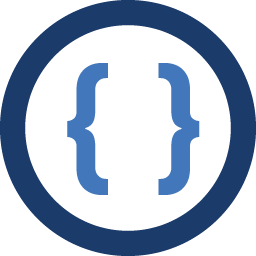
Admin
Updated on March 01, 2020Comments
-
Admin about 4 years
I want to know how to get remainder and quotient in single value in Java.
Example:
3/2 I should get value as 1.5.
If I use the
/
operator I get only the quotient. If I user the%
operator I get only the remainder. How do I get both at a time in the same variable?