How do I encrypt and decrypt a string in python?
Solution 1
I had troubles compiling all the most commonly mentioned cryptography libraries on my Windows 7 system and for Python 3.5.
This is the solution that finally worked for me.
from cryptography.fernet import Fernet
key = Fernet.generate_key() #this is your "password"
cipher_suite = Fernet(key)
encoded_text = cipher_suite.encrypt(b"Hello stackoverflow!")
decoded_text = cipher_suite.decrypt(encoded_text)
Solution 2
Take a look at PyCrypto. It supports Python 3.2 and does exactly what you want.
From their pip website:
>>> from Crypto.Cipher import AES
>>> obj = AES.new('This is a key123', AES.MODE_CFB, 'This is an IV456')
>>> message = "The answer is no"
>>> ciphertext = obj.encrypt(message)
>>> ciphertext
'\xd6\x83\x8dd!VT\x92\xaa`A\x05\xe0\x9b\x8b\xf1'
>>> obj2 = AES.new('This is a key123', AES.MODE_CFB, 'This is an IV456')
>>> obj2.decrypt(ciphertext)
'The answer is no'
If you want to encrypt a message of an arbitrary size use AES.MODE_CFB
instead of AES.MODE_CBC
.
Solution 3
Try this:
Python Cryptography Toolkit (pycrypto) is required
$ pip install pycrypto
pycrypto
package is outdated and has not been maintained since 2014.
There is a drop-in replacement package called pycryptodome
.
$ pip install pycryptodome
And the code below flawlessly works on python 3.8
Code:
from Crypto.Cipher import AES
from base64 import b64encode, b64decode
class Crypt:
def __init__(self, salt='SlTKeYOpHygTYkP3'):
self.salt = salt.encode('utf8')
self.enc_dec_method = 'utf-8'
def encrypt(self, str_to_enc, str_key):
try:
aes_obj = AES.new(str_key.encode('utf-8'), AES.MODE_CFB, self.salt)
hx_enc = aes_obj.encrypt(str_to_enc.encode('utf8'))
mret = b64encode(hx_enc).decode(self.enc_dec_method)
return mret
except ValueError as value_error:
if value_error.args[0] == 'IV must be 16 bytes long':
raise ValueError('Encryption Error: SALT must be 16 characters long')
elif value_error.args[0] == 'AES key must be either 16, 24, or 32 bytes long':
raise ValueError('Encryption Error: Encryption key must be either 16, 24, or 32 characters long')
else:
raise ValueError(value_error)
def decrypt(self, enc_str, str_key):
try:
aes_obj = AES.new(str_key.encode('utf8'), AES.MODE_CFB, self.salt)
str_tmp = b64decode(enc_str.encode(self.enc_dec_method))
str_dec = aes_obj.decrypt(str_tmp)
mret = str_dec.decode(self.enc_dec_method)
return mret
except ValueError as value_error:
if value_error.args[0] == 'IV must be 16 bytes long':
raise ValueError('Decryption Error: SALT must be 16 characters long')
elif value_error.args[0] == 'AES key must be either 16, 24, or 32 bytes long':
raise ValueError('Decryption Error: Encryption key must be either 16, 24, or 32 characters long')
else:
raise ValueError(value_error)
Usage:
test_crpt = Crypt()
test_text = """Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam,
quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo
consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse
cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non
proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam,
quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo
consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse
cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non
proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam,
quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo
consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse
cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non
proident, sunt in culpa qui officia deserunt mollit anim id est laborum."""
test_key = 'MyKey4TestingYnP'
test_enc_text = test_crpt.encrypt(test_text, test_key)
test_dec_text = test_crpt.decrypt(test_enc_text, test_key)
print(f'Encrypted:{test_enc_text} Decrypted:{test_dec_text}')
Solution 4
You can do this easily by using the library cryptocode
. Here is how you install:
pip install cryptocode
Encrypting a message (example code):
import cryptocode
encoded = cryptocode.encrypt("mystring","mypassword")
## And then to decode it:
decoded = cryptocode.decrypt(encoded, "mypassword")
Documentation can be found here
Solution 5
Encrypt Data
First, we need to install the cryptography library:
pip3 install cryptography
-
From the cryptography library, we need to import
Fernet
and start generating a key - this key is required for symmetric encryption/decryption. -
To generate a key, we call the
generate_key()
method.- We only need to execute the above method once to generate a key.
You need to keep this key in a safe place. If you lose the key, you won't be able to decrypt the data that was encrypted with this key.
-
Once we have generated a key, we need to load the key with
load_key()
Encrypt a Message
This is a three step process:
- encode the message
- initialize the Fernet class
- pass the encoded message to
encrypt()
method
Below is a full working example of encrypting a message :
from cryptography.fernet import Fernet
def generate_key():
"""
Generates a key and save it into a file
"""
key = Fernet.generate_key()
with open("secret.key", "wb") as key_file:
key_file.write(key)
def load_key():
"""
Load the previously generated key
"""
return open("secret.key", "rb").read()
def encrypt_message(message):
"""
Encrypts a message
"""
key = load_key()
encoded_message = message.encode()
f = Fernet(key)
encrypted_message = f.encrypt(encoded_message)
print(encrypted_message)
if __name__ == "__main__":
# generate_key() # execute only once
encrypt_message("Hello stackoverflow!")
output:
b'gAAAAABgLX7Zj-kn-We2BI_c9NQhEtfJEnHUVhVqtiqjkDi5dgJafj-_8QUDyeNS2zsJTdBWg6SntRJOjOM1U5mIxxsGny7IEGqpVVdHwheTnwzSBlgpb80='
Decrypt Data
To decrypt the message, we just call the decrypt()
method from the Fernet
library. Remember, we also need to load the key as well, because the key is needed to decrypt the message.
from cryptography.fernet import Fernet
def load_key():
"""
Load the previously generated key
"""
return open("secret.key", "rb").read()
def decrypt_message(encrypted_message):
"""
Decrypts an encrypted message
"""
key = load_key()
f = Fernet(key)
decrypted_message = f.decrypt(encrypted_message)
print(decrypted_message.decode())
if __name__ == "__main__":
decrypt_message(b'gAAAAABgLX7Zj-kn-We2BI_c9NQhEtfJEnHUVhVqtiqjkDi5dgJafj-_8QUDyeNS2zsJTdBWg6SntRJOjOM1U5mIxxsGny7IEGqpVVdHwheTnwzSBlgpb80=')
output:
Hello stackoverflow!
Your password is in the secret.key
in a form similar to the password below:
B8wtXqwBA_zb2Iaz5pW8CIQIwGSYSFoBiLsVz-vTqzw=
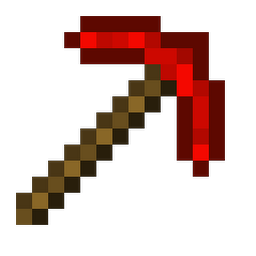
David
Updated on July 05, 2022Comments
-
David almost 2 years
I have been looking for sometime on how to encrypt and decrypt a string. But most of it is in 2.7 and anything that is using 3.2 is not letting me print it or add it to a string.
So what I'm trying to do is the following:
mystring = "Hello stackoverflow!" encoded = encode(mystring,"password") print(encoded)
jgAKLJK34t3g (a bunch of random letters)
decoded = decode(encoded,"password") print(decoded)
Hello stackoverflow!
Is there anyway of doing this, using python 3.X and when the string is encoded it's still a string, not any other variable type.
-
David over 9 yearsWhen I try import it it gives me an error: >>> from Crypto.Cipher import AES Traceback (most recent call last): File "<pyshell#0>", line 1, in <module> from Crypto.Cipher import AES File "C:\Python34\lib\Crypto\Cipher\AES.py", line 50, in <module> from Crypto.Cipher import _AES ImportError: cannot import name '_AES' >>> Did I install it right? I downloaded Crypto and copied the folder which is in the lib folder into the Lib folder in pythons directory.
-
Piotr Dabkowski over 9 yearsCopying sometimes is not enough. Use python setup.py install or type pip install pycrypto in your command line. You can download pip here: lfd.uci.edu/~gohlke/pythonlibs/#pip
-
SanD over 8 yearsIn case u need to encrypt a message of arbitrary size other than size n*16 byte, u can use AES.MODE_CFB instead of MODE_CBC. obj = AES.new('This is a key123', AES.MODE_CFB, 'This is an IV456'). Refer the docs at pythonhosted.org/pycrypto
-
Rohit Khatri over 7 yearsI'm getting this error while encoding
'utf-8' codec can't decode byte 0xa6 in position 7: invalid start byte
-
rahul.m about 7 yearshow pass a variable in encoded_text = cipher_suite.encrypt(b(pk))
-
KRBA about 7 years@stone, you just need to convert text to a byte stream: encoding = "utf-8" #for example pk = bytes(original_text, encoding) encoded_text = cipher_suite.encrypt(pk)
-
ADL about 7 yearsError: Input strings must be a multiple of 16 in length.
-
Ankur Sharma over 6 years@RohitKhatri Did you get the solution to your error ?
-
Brōtsyorfuzthrāx over 5 yearsAny way to make your own key text?
-
Maddie Graham about 5 yearsI had the same problem. You are saving my life. Thank you!
-
Naveen Reddy Marthala over 4 yearsi have just copy pasted your code and did nothing more. my error is:
TypeError: Object type <class 'str'> cannot be passed to C code
-
default123 over 3 yearsWhy does this generate a different value everytime you run
cipher_suite.encrypt()
? -
Tatarize over 3 yearscipher_suite = Fernet(base64.urlsafe_b64encode(b'12345678901234567890123456789012'))
-
Sanjay Sikdar over 2 yearsCan you tell me how can I use it with Django.? What I have tried: I'm storing the encrypted message on the database. after that, I'm trying to decrypt that message and getting
token must be bytes
error! -
Milovan Tomašević over 2 yearsI think that in the first step you should have:
cript_body_message = decipher.decrypt(form.message.data).decode('utf-8')
this will encrypt the message and prepare it for storage. After that, you probably create an object withmessage = Message(header=form.header.data, body=cript_body_message)
. And finally adding to the databasedb.session.add(message)
anddb.session.commit()
. Something personal to him should do. It should be noted that when reading, it is necessary to decipher the message. I hope I helped at least a little. -
Milovan Tomašević over 2 yearsYou should also look at: django-crypto-fields, django-cryptographic-fields, django-cryptography ... The documentations is excellent as are the examples. Happy coding (:
-
Mike over 2 yearsIs reusing
cipher_suite
for other strings safe? -
Cagatay Barin over 2 yearsIf you're using python 3, use the pycryptodome package with the same code in this answer. It works for me on python 3.8 , so I've modified the answer (including a minor bugfix in the code)
-
Shreyansh Dwivedi over 2 yearswhen I run your code it gives error -> AttributeError: module 'hashlib' has no attribute 'scrypt'