How do I execute Windows commands in Java?
Solution 1
I hope this helps :)
You could use:
Runtime.getRuntime().exec("ENTER COMMAND HERE");
Solution 2
Take advantage of the ProcessBuilder
.
It makes it easier to build the process parameters and takes care of issues with having spaces in commands automatically...
public class TestProcessBuilder {
public static void main(String[] args) {
try {
ProcessBuilder pb = new ProcessBuilder("cmd", "/c", "dir");
pb.redirectError();
Process p = pb.start();
InputStreamConsumer isc = new InputStreamConsumer(p.getInputStream());
isc.start();
int exitCode = p.waitFor();
isc.join();
System.out.println("Process terminated with " + exitCode);
} catch (IOException | InterruptedException exp) {
exp.printStackTrace();
}
}
public static class InputStreamConsumer extends Thread {
private InputStream is;
public InputStreamConsumer(InputStream is) {
this.is = is;
}
@Override
public void run() {
try {
int value = -1;
while ((value = is.read()) != -1) {
System.out.print((char)value);
}
} catch (IOException exp) {
exp.printStackTrace();
}
}
}
}
I'd generally build a all purpose class, which you could pass in the "command" (such as "dir") and it's parameters, that would append the call out to the OS automatically. I would also included the ability to get the output, probably via a listener callback interface and even input, if the command allowed input...
Solution 3
This is a sample code to run and print the output of the ipconfig command in the console window.
import java.io.IOException;
import java.io.InputStream;
public class ExecuteDOSCommand {
public static void main(String[] args) {
final String dosCommand = "ipconfig";
try {
final Process process = Runtime.getRuntime().exec(dosCommand );
final InputStream in = process.getInputStream();
int ch;
while((ch = in.read()) != -1) {
System.out.print((char)ch);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Source: https://www.codepuran.com/java/execute-dos-command-java/
Solution 4
Old question but might help someone passing by. This is a simple and working solution. Some of the above solutions don't work.
import java.io.IOException;
import java.io.InputStream;
public class ExecuteDOSCommand
{
public static void main(String[] args)
{
final String dosCommand = "cmd /c dir /s";
final String location = "C:\\WINDOWS\\system32";
try
{
final Process process = Runtime.getRuntime().exec(dosCommand + " " + location);
final InputStream in = process.getInputStream();
int ch;
while((ch = in.read()) != -1)
{
System.out.print((char)ch);
}
}
catch (IOException e)
{
e.printStackTrace();
}
}
}
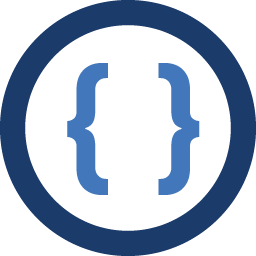
Admin
Updated on December 29, 2020Comments
-
Admin over 3 years
I'm working on a project, and it will give you a list of Windows commands. When you select one, it will perform that command. However, I don't know how to do that. I was going to do it in Visual C#, or C++, but C++ classes are too complicated, and I don't want to make the forms and junk in Visual C# (really bad at console applications).