How do I filter out a string to contain only letters in vanilla Javascript?
A simple way may be to use Regex like so
let message = "Learning is fun!";
let onlyLettersArray = message.split('').filter(char => /[a-zA-Z]/.test(char));
console.log(onlyLettersArray)
.filter takes an array and runs a function on the elements, which returns true or false. The item is removed if it returns false. The regex checks if the character is within the range a-z or A-Z
Another way is to filter the char and then split it like so
let message = "Learning is fun!";
let onlyLettersArray = message.replace(/[^a-z]+/gi, '').split('');
console.log(onlyLettersArray)
Edit:
var myMessage = "Learning is fun!";
var arr1 = myMessage.split("");
function onlyLetters(array){
let arr2 = []
for(let i = 0; i < array.length; i++){
if(/[a-z]/.test(array[i])){ // you can use regex instead of all characters
arr2.push(array[i])
}
}
return arr2
}
console.log(onlyLetters(myMessage))
Update: If instead of an array of characters, you have to replace special chars in a string, you can write
let message = "Learning is fun!";
let letterMessage = message.replace(/[^a-zA-Z]/gm,"")
console.log(letterMessage)
Related videos on Youtube
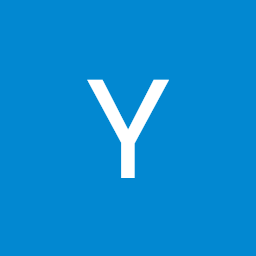
Yumi Park
Currently a student at LEARN Academy learning Javascript and Ruby. I'm new to programming and I'm excited to go further into my programming journey.
Updated on May 25, 2022Comments
-
Yumi Park almost 2 years
I was given this string:
var myMessage = "Learning is fun!"
This is how I attempted to create an array listing only the letters (without the spaces and "!").
var myMessage = "Learning is fun!"; var arr1 = myMessage.split(""); function onlyLetters(array){ let arr2 = [] for(let i = 0; i < array.length; i++){ if(array[i] === "a" || "b" || "c" || "d" || "e" || "f" || "g" || "h" || "i" || "j" || "k" || "l" || "m" || "n" || "o" || "p" || "q" || "r" || "s" || "t" || "u" || "v" || "w" || "x" || "y" || "z"){ arr2.push(array[i]) } } return arr2 } console.log(onlyLetters(myMessage))
What am I doing wrong? Also, is there a shorthand for listing letters "a" through "z"?
-
ninesalt over 4 yearsYou can't do
array[i] === "a" || "b" || ....
because the right side of the===
is evaluated as"a" || "b" || ....
. What you mean to do isarray[i] === "a" || array[i] === "b" || ...
-
-
ninesalt over 4 yearsI'd argue that since OP is a beginner, it is more helpful to them to help with their existing code rather than give them something new.
-
Dhananjai Pai over 4 yearsI have given the explanation as well. I believe it may be a good way for them to learn the features javascript can offer as well. But you do have a valid point and I will update the code to add what the OP has used
-
Dhananjai Pai over 4 yearsI think he wanted an array of characters based on the question. If it is a string, you could replace the special chars @AZ_
-
Dhananjai Pai over 4 yearsHe hasn't used a join() in the end
-
ninesalt over 4 yearsYou can also do
message.replace(/[^a-z]+/gi, '').split('')
-
Dhananjai Pai over 4 yearsit is String.prototype.match. Not array
-
AZ_ over 4 years@DhananjaiPai oops! typo
-
Dhananjai Pai over 4 yearsyeah @ninesalt that makes more sense too