How do I generate a hash code with hash sha256 in java?
Solution 1
I'm still unclear whether you want SHA-1 or SHA-256, so let's abstract the problem; firstly, an encode method to take a byte[]
and return the hex (don't worry, you already wrote it; but I would prefer a StringBuilder
over String
concatenation. Java String
is immutable, so you're creating garbage for later garbage collection with +
) -
private static String encodeHex(byte[] digest) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < digest.length; i++) {
sb.append(Integer.toString((digest[i] & 0xff) + 0x100, 16).substring(1));
}
return sb.toString();
}
Next, we can create a method that takes the algorithm name and the String
to digest and returns that digest. Like
public static String digest(String alg, String input) {
try {
MessageDigest md = MessageDigest.getInstance(alg);
byte[] buffer = input.getBytes("UTF-8");
md.update(buffer);
byte[] digest = md.digest();
return encodeHex(digest);
} catch (NoSuchAlgorithmException | UnsupportedEncodingException e) {
e.printStackTrace();
return e.getMessage();
}
}
Then we can get a SHA-1
or a SHA-256
hash like
public static void main(String[] args) {
System.out.println(digest("SHA1", ""));
System.out.println(digest("SHA-256", ""));
}
Which outputs (as expected)
da39a3ee5e6b4b0d3255bfef95601890afd80709
e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855
Solution 2
The main entry point can not return String. Furthermore, input
is not declared. You maybe want to change the name of your function to generate
with input
as a parameter.
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import static jdk.nashorn.tools.ShellFunctions.input;
public class Sha256hash
{
public static String generate(String input) throws NoSuchAlgorithmException
{
MessageDigest md = MessageDigest.getInstance("SHA1");
md.reset();
byte[] buffer = input.getBytes("UTF-8");
md.update(buffer);
byte[] digest = md.digest();
String hexStr = "";
for (int i = 0; i < digest.length; i++) {
hexStr += Integer.toString( ( digest[i] & 0xff ) + 0x100, 16).substring( 1 );
}
return hexStr;
}
}
This example works for me returning c3499c2729730a7f807efb8676a92dcb6f8a3f8f
as result of processing the string example
:
import java.io.UnsupportedEncodingException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class Sha256hash
{
public static String generate(String input) throws NoSuchAlgorithmException, UnsupportedEncodingException
{
MessageDigest md = MessageDigest.getInstance("SHA1");
md.reset();
byte[] buffer = input.getBytes("UTF-8");
md.update(buffer);
byte[] digest = md.digest();
String hexStr = "";
for (int i = 0; i < digest.length; i++) {
hexStr += Integer.toString( ( digest[i] & 0xff ) + 0x100, 16).substring( 1 );
}
return hexStr;
}
}
Main:
import java.io.UnsupportedEncodingException;
import java.security.NoSuchAlgorithmException;
public class Tester {
public static void main(String[] args) throws NoSuchAlgorithmException, UnsupportedEncodingException {
String someText = "example";
System.out.println(Sha256hash.generate(someText));
}
}
Finally, as Elliott has pointed out If you want to use SHA-256 you should change MessageDigest.getInstance("SHA1");
to MessageDigest.getInstance("SHA256")
; Right now you are using SHA-1. Also pointed by Elliot you should use StringBuilder
in the loop for improved efficiency.
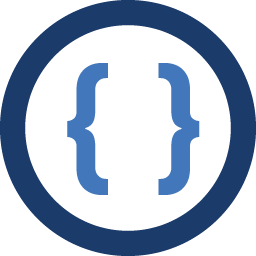
Admin
Updated on June 28, 2022Comments
-
Admin almost 2 years
I would like to know the code to do this in java please?
This is what i have so far but it does not work?
import java.security.MessageDigest; import java.security.NoSuchAlgorithmException; import static jdk.nashorn.tools.ShellFunctions.input; public class Sha256hash { public static String main(String[] args) throws NoSuchAlgorithmException { MessageDigest md = MessageDigest.getInstance("SHA1"); md.reset(); byte[] buffer = input.getBytes("UTF-8"); md.update(buffer); byte[] digest = md.digest(); String hexStr = ""; for (int i = 0; i < digest.length; i++) { hexStr += Integer.toString( ( digest[i] & 0xff ) + 0x100, 16).substring( 1 ); } return hexStr; } }
-
nico over 4 yearsI think the digest fonction can be dangerous as it is, it return a string even if the algo is unknown/wrongly typed. If someone want to use this code, I would recommend to not catch execeptions here.