how do i get a bearer token in a .Net console application?
Solution 1
I'm not able to test this myself right now, but I think you've missed out some text in your authCredentials
. It should look like this:
var authCredentials = "grant_type=password&userName=" + user + "&password=" + password;
Note the additional: grant_type=password& that I added at the beginning. This updated whole string authCredentials
should be the only thing in your request body.
Assuming the request succeeds and it doesn't fail for other reasons, e.g. your username or password is not valid, then you should be able to get back your token data.
Also, the response will contain the access_token
in a json-formatted string in its body, not in a cookie header where you're reading it from.
Solution 2
FormUrlEncodedContent is in System.Net.Http
static string GetToken(string userName, string password)
{
var pairs = new List<KeyValuePair<string, string>>
{
new KeyValuePair<string, string>( "grant_type", "password" ),
new KeyValuePair<string, string>( "userName", userName ),
new KeyValuePair<string, string> ( "password", password )
};
var content = new FormUrlEncodedContent(pairs);
using (var client = new HttpClient())
{
var response =
client.PostAsync("https://localhost:12698/Token", content).Result;
return response.Content.ReadAsStringAsync().Result;
}
}
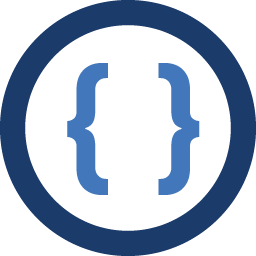
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I was following this tutorial about how to authorize and get a bearer token from a web-api server. The tasks performed there through fiddler are pretty simple, but, when I tried to do the same from a console application the request fails with a
400 Bad Request Error
. Here is the code that makes the request to the server:var request = WebRequest.Create("http://localhost:12698/token") as HttpWebRequest; request.Method = "POST"; request.ContentType = "application/x-www-form-urlencoded"; request.CookieContainer = new CookieContainer(); var authCredentials = "userName=" + user + "&password=" + password; byte[] bytes = System.Text.Encoding.UTF8.GetBytes(authCredentials); request.ContentLength = bytes.Length; using (var requestStream = request.GetRequestStream()){ requestStream.Write(bytes, 0, bytes.Length); } using (var response = request.GetResponse() as HttpWebResponse){ authCookie = response.Cookies["access_token"]; }
can someone help me in determining what I am doing wrong here? or is there some other approach I should use to get the authentication token?
-
Admin almost 10 yearsI had tried that earlier but got an empty cookie header when i got the response. The mistake I was doing was indeed looking for the token the wrong place (its in the response body, not in the cookie header). Thanks for pointing that out for me.