How do I get a list of fields returned by an OdbcDataReader?
Solution 1
I think I know what you may be looking for. If you are asking "Regardless of the query text can you list the field names of the returned dataset without hitting the db for a schema check?" then this should work for you:
var db = new System.Data.Odbc.OdbcConnection ( @"MyODBCSqlConnectionString" );
db.Open();
var cmd = db.CreateCommand();
cmd.CommandText = "SELECT * from MyTable";
var reader = cmd.ExecuteReader();
for( int ordinal = 0; ordinal< reader.FieldCount; ordinal++)
Console.WriteLine( "Field {0}: {1}", ordinal, reader.GetName( ordinal ) );
Solution 2
You can use OdbcDataReader.GetSchemaTable
to get information about the columns for the current result set (I think - the documentation isn't clear about whether or not it supports multiple result sets). You should read the linked documentation if you plan to rely on this method.
In particular, note the following:
The .NET Framework Data Provider for ODBC assumes that metadata information is available from an ODBC driver after one of SQLPrepare, SQLExecute, or SQLExecuteDirect functions are called. For "SchemaOnly" command behavior to work correctly, SQLPrepare must return the required metadata information. Not all ODBC drivers support this function or return metadata information.
And this:
To make sure that metadata columns return the correct information, you must call ExecuteReader with the behavior parameter set to KeyInfo. Otherwise, some of the columns in the schema table may return default, null, or incorrect data.
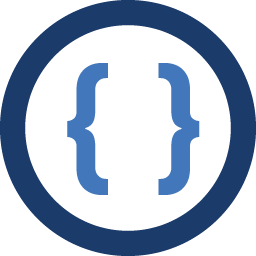
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
How can I get a list of fields from OdbcDataReader in C#?
For example, for a table with two fields,
Field1
andField2
:DbCommand.CommandText = "SELECT * FROM TABLE WHERE 0=1"; DbReader = DbCommand.ExecuteReader();
DbReader.???
would give to me a list of two elements -["Field1","Field2"]
Is there such a function?