How do I get a listing of only files using Dir.glob?
Solution 1
In addition to Mark's answer, Dir.entries
will give back directories. If you just want the files, you can test each entry to see if it's a file or a directory, by using file?
.
Dir.entries('/home/theiv').select { |f| File.file?(f) }
Replace /home/theiv
with whatever directory you want to look for files in.
Also, have a look at File. It provides a bunch of tests and properties you can retrieve about files.
Solution 2
Dir.glob('*').select { |fn| File.file?(fn) }
Solution 3
Entries don't do rescursion i think. If you want the files in the subdirs also use
puts Dir['**/*'].select { |f| File.file?(f) }
Solution 4
If you want to do it in one go instead of first creating an array and then iterating over it with select
, you can do something like:
my_list = []
Dir.foreach(dir) { |f| my_list << f if File.file?(f) }
Solution 5
Following @karl-li 's suggestion in @theIV solution, I found this to work well:
Dir.entries('path/to/files/folder').reject { |f| File.directory?(f) }
Related videos on Youtube
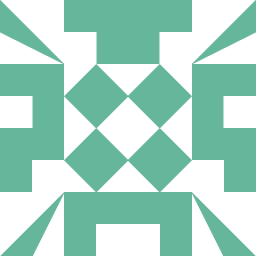
user982570
Updated on June 04, 2022Comments
-
user982570 12 months
How can I return a list of only the files, not directories, in a specified directory?
I have
my_list = Dir.glob(script_path.join("*"))
This returns everything in the directory,including subdirectories. I searched but haven't been able to find the answer.
-
PJP over 11 years
Dir.entries
returns both files and subdirectories, and is similar toglob
without the filename mask. ` Dir.entries("testdir") #=> [".", "..", "config.h", "main.rb"]`. The first two values returned are directories. -
Aram Kocharyan about 10 yearsI had to use
File.file?("/home/theiv/#{f}")
-
karl li about 6 yearsin the mapping section: select {|f| File.file?(f)}, the |f| is just the file name, not complete path. So you need to compose a complete one, say File.file?("/home/theiv/#{f}"), otherwise it doesn't work. Or for a simpler way, use: !File.directory(f) instead.