How do I get DeviceOrientationEvent and DeviceMotionEvent to work on Safari?
Solution 1
if ( location.protocol != "https:" ) {
location.href = "https:" + window.location.href.substring( window.location.protocol.length );
}
function permission () {
if ( typeof( DeviceMotionEvent ) !== "undefined" && typeof( DeviceMotionEvent.requestPermission ) === "function" ) {
// (optional) Do something before API request prompt.
DeviceMotionEvent.requestPermission()
.then( response => {
// (optional) Do something after API prompt dismissed.
if ( response == "granted" ) {
window.addEventListener( "devicemotion", (e) => {
// do something for 'e' here.
})
}
})
.catch( console.error )
} else {
alert( "DeviceMotionEvent is not defined" );
}
}
const btn = document.getElementById( "request" );
btn.addEventListener( "click", permission );
Use an element on your page to use as the event trigger and give it an id of "request".
This will check for https and change it if required before requesting API authorization. Found this yesterday but do not remember the URL.
Solution 2
As of iOS 13 beta 2, you need to call DeviceOrientationEvent.requestPermission() to access to gyroscope or accelerometer. This will present a permission dialog prompting the user to allow motion and orientation access for this site.
Note that this will not work if you try to call it automatically when the page loads. The user needs to take some action (like tapping a button) to be able to display the dialog.
Also, the current implementation seems to require that the site have https enabled.
For more information, see this page
Solution 3
You need a click or a user gesture to call the requestPermission(). Like this :
<script type="text/javascript">
function requestOrientationPermission(){
DeviceOrientationEvent.requestPermission()
.then(response => {
if (response == 'granted') {
window.addEventListener('deviceorientation', (e) => {
// do something with e
})
}
})
.catch(console.error)
}
</script>
<button onclick='requestOrientationPermission();'>Request orientation permission</button>
Note : if you click on cancel on the permission prompt and want to test it again, you will need to quit Safari and launch it back for the prompt to come back.
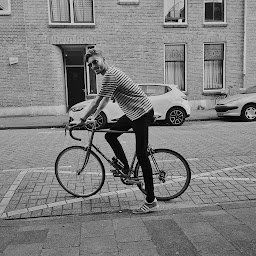
Siemen Gijbels
Updated on June 13, 2022Comments
-
Siemen Gijbels almost 2 years
I'm trying to implement DeviceOrientationEvent and DeviceMotionEvent on my website for a 3D effect. However, the console doesn't log any info and apparently iOS 13 requires a user set permission to start doing this. I can't seem to figure out how to set it up properly.
I've done some research and this is what I found: https://github.com/w3c/deviceorientation/issues/57#issuecomment-498417027
All other methods provided online are not usable anymore sadly.
window.addEventListener('deviceorientation', function(event) { console.log(event.alpha + ' : ' + event.beta + ' : ' + event.gamma); });
I get the following error message:
[Warning] No device motion or orientation events will be fired until permission has been requested and granted.
-
FireFuro99 over 2 yearsThis alerts "DeviceMotionEvent is not defined" in my Safari Browser on my iPhone 6S (iOS 12). Why doesn't it work?