How do I get from the "Event" object the object on which there was this event?
Solution 1
You have to cast the source object to the object you expect. The getSource() method only delivers you an object, but you can't access any information from it (e.g. from a Button, you first have to cast it to a button).
Here is an example:
Button bt = new Button("click");
bt.addClickHandler(new ClickHandler() {
@Override
public void onClick(ClickEvent event) {
Object soruce = event.getSource();
if (soruce instanceof Button) { //check that the source is really a button
String buttonText = ((Button) soruce).getText(); //cast the source to a button
RootPanel.get().add(new Label(buttonText));
} else {
RootPanel.get().add(new Label("Not a Button, can't be..."));
}
}
});
RootPanel.get().add(bt);
This of course also works for UiBinder Buttons:
@UiHandler("button")
void onClick(ClickEvent e) {
Object soruce = e.getSource();
if(soruce instanceof Button){
String buttonText = ((Button)soruce).getText();
RootPanel.get().add(new Label(buttonText));
}
else {
RootPanel.get().add(new Label("The event is not bound to a button"));
}
}
If you don't know the type of your element, or the event is bound to more than one element, you have to check for all possible types first, and then perform the right action.
Solution 2
If you want one method to handle click events for a bunch of widgets, then it's as easy as:
@UiHandler({ "firstWidget", "secondWidget", "thirdWidget", "fourthWidget", andSoOn" })
void universalClickHandler(ClickEvent event) {
// here, you can use event.getSource() to get a reference to the actual widget
// that the event targeted.
}
If you want to use event delegation over a bunch of elements, then you need to listen to click events on an ancestor, and then you can use event.getNativeEvent().getEventTarget()
to get the actual element that was the target of the click. You can then use isOrHasChild
on an Element
to know whether the actual target of the click was within that element (e.g. firstElement.isOrHasChild(Element.as(event.getNativeEvent().getEventtarget()))
, with firstElement
being a @UiField Element
–or any subclass or Element
, such as DivElement
)
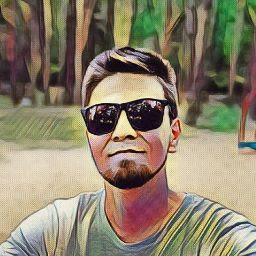
Hleb Kastseika
Updated on June 04, 2022Comments
-
Hleb Kastseika almost 2 years
I am developing an application on GWT with UIBinder and I have a problem. In my user interface can be a lot of the same elements (eg widgets). All elements must have handlers that will catch the mouse click event. I want to write a universal handler that will be able to identify the widget that caused the event and handle it instead. Now I have a widget for each object to describe the same handler. How can I solve this problem?