How do I link auth users to collection in Firestore?
Solution 1
Right now what i have done is when a user creates an account
i will log the login information into the database.
The document name is set to the user UID that firebase give the user.
Now you can simply request the data from the database with the user UID as
being your .doc(user.uid)
.
This is the full code.
var htmlEmail = document.getElementById('email').value;
var htmlPass = document.getElementById('password').value;
var htmlUser = document.getElementById('username').value.toLowerCase();
var auth = firebase.auth();
var promise = auth.createUserWithEmailAndPassword(htmlEmail, htmlPass);
// If there is any error stop the process.
promise.catch(function (error) {
var errorCode = error.code;
console.log(`GOT ERROR: ` + errorCode)
if (errorCode == 'auth/weak-password') return // password to weak. Minimal 6 characters
if (errorCode == 'auth/email-already-in-use') return // Return a email already in use error
});
// When no errors create the account
promise.then(function () {
var userUid = auth.currentUser.uid;
var db = firebase.firestore();
db.collection('users').doc(userUid).set({
email: htmlEmail,
emailVertified: false,
name: htmlUser,
online: false,
onlock: false,
password: htmlPass
});
Then when the user logs you can simply request the data over the user.uid.
var auth = firebase.auth();
firebase.auth().onAuthStateChanged(function (user) {
// Lay connection with the database.
var firestore = firebase.firestore();
var db = firestore.collection('users').doc(user.uid);
// Get the user data from the database.
db.get().then(function (db) {
// Catch error if exists.
promise.catch(function (error) {
// Return error
});
promise.then(function () {
// continue when success
});
});
});
There could just be there are better ways. (still learning myself). But this does the trick for me and works very well.
There are 2 things to keep in mind !
- I would recommend Firestore over the real time database as it is faster and more secure.
- Make sure your database rules are set correctly, so that no one can view / leak your database information. (as you are logging users personal info). If not set correctly users will be able to view your database and even purge all data.
Hope it helps :)
If you find a better way yourself please let us know in here. We could learn from that also !
Solution 2
In a simplified way you can do this, everytime a user will signup this function will create a firestore collection with the specific parameters.
signupWithEmail: async (_, { email, password, name }) => {
var user = firebase.auth().createUserWithEmailAndPassword(email,
password).then(cred => {
return
firebase.firestore().collection('USERS').doc(cred.user.uid).set({
email,
name
})
})
return { user }
}
Related videos on Youtube
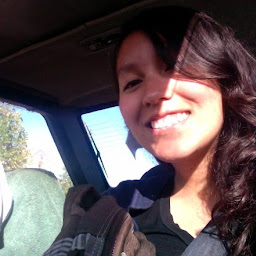
Rachele Avella
Updated on June 04, 2022Comments
-
Rachele Avella almost 2 years
I'm trying to connect a user to the user collection in firestore. I'm using cloud functions, but I don't think I'm implementing it correctly.
firebase.auth().createUserWithEmailAndPassword(email, password) .then(() => { console.log('user created') exports.createUserDoc = functions.auth.user().onCreate((user) => { console.log("hi") const userId = user.uid; const account = { posts: [] } return admin.firestore().collection("Users").doc(userId).add(account) })
But my console.log(hi) isn't showing up. Am I approaching this correctly? Any advice helps!
-
Ziyo over 5 yearsI think you need to deploy your cloud function first. That function gets triggered automatically when you create a user. You do not implement cloud function inside your app. cloud.google.com/functions/docs/quickstart-console
-
-
ElKePoN over 3 yearsWhy would you store the password into the database? That doesn't sound good, I would rather leave it encrypted where it is, you don't need the password for anything in firestore
-
Ali Shirazee over 3 yearsHey, the snippet is from here. firebase.google.com/docs/auth/web/password-auth. The password is not saved to the database.
-
Ali Shirazee over 3 yearshave a look at this Gist: gist.github.com/trackmystories/3694dafb93f367359864f7824cd3c57b
-
Ali Shirazee over 3 yearsessentially what this code is saying is var user = create a user with email and password with the parameters email and password provided to your form, and then set the email and the name not the password in the firebase.firestore collections document with a user uid. It would be mal practice if the password was saved in firebase.firestore().collection('USERS').doc(cred.user.uid).set({ email, name })
-
Ali Shirazee over 3 yearsI would highly suggest reading firebase.google.com/docs/auth/web/manage-users to get an in-depth understanding on how users are managed and for password authentication read this firebase.google.com/docs/auth/web/password-auth