how do i program the youtube embed player to unmute when clicked
Solution 1
Thank you for that answer! I implemented it a little differently, adding it to the onYouTubeIframeAPIReady function.
I also reversed the initialized value of the isUnMuted variable. Functionally it is the same, but makes more sense this way.
var player;
var isUnMuted = false;
window.onYouTubeIframeAPIReady = function() {
player = new YT.Player('youTube', {
videoId: '[myVideoId]',
playerVars: {
'rel': 0,
},
events: {
'onReady': function() {
player.mute();
player.playVideo();
},
'onStateChange': function () {
if (player.isMuted() && player.getPlayerState() == 2 && !isUnMuted) {
player.unMute();
player.playVideo();
isUnMuted = true;
}
}
}
});
}
This mimics closely the behavior of embedded videos in a Facebook newsfeed.
Solution 2
I don't think it is possible, because there is not such thing as a 'click' or 'activate' event. However, you may try adding an event handler to the "onStateChange" event and unmute and unpause your player. I haven't tried it, but give it a try and see if this works:
var isUnMuted = true;
player.addEventListener("onStateChange", function(event) {
if( player.isMuted() && player.getPlayerState() == 2 && isUnMuted ) {
player.unMute();
player.playVideo(); // resume playback
isUnMuted = false; // you want to run this once only!
}
});
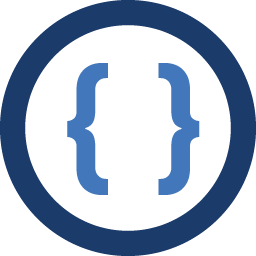
Admin
Updated on June 12, 2022Comments
-
Admin almost 2 years
How do I set the youtube embedded player to unmute upon clicking it. You can see the embedded player i'm referring to at http://www.harvestarmy.org home page. It's the one at the right where it says "Latest Videos from YouTube. I set it up to be muted by default, but I want it to automatically unmute when someone clicks it.
Here is the code I used:
<!-- 1. The <iframe> (and video player) will replace this <div> tag. --> <div id="player"></div> <script> // 2. This code loads the IFrame Player API code asynchronously. var tag = document.createElement('script'); // This is a protocol-relative URL as described here: // http://paulirish.com/2010/the-protocol-relative-url/ // If you're testing a local page accessed via a file:/// URL, please set tag.src to // "https://www.youtube.com/iframe_api" instead. tag.src = "//www.youtube.com/iframe_api"; var firstScriptTag = document.getElementsByTagName('script')[0]; firstScriptTag.parentNode.insertBefore(tag, firstScriptTag); // 3. This function creates an <iframe> (and YouTube player) // after the API code downloads. var player; function onYouTubeIframeAPIReady() { player = new YT.Player('player', { height: '98', width: '175', playerVars: { 'list': 'UUFwY5Al1Doy7f0MdKnJ-gaw', 'modestbranding': 1, 'showinfo': 0, 'autoplay': 1 }, events: { 'onReady': onPlayerReady } }); } // 4. The API will call this function when the video player is ready. function onPlayerReady(event) { event.target.mute(); } </script>