How do I PUT data to Rails using JQuery
Solution 1
PUT and DELETE are not supported by all browsers, RubyOnRails supports passing an extra parameter with your data called _method which will indicate how RoR will treat the request.
$.ajax({
type: "POST",
url: '/admin/pages/1.json',
data: { _method:'PUT', page : {...} },
dataType: 'json',
success: function(msg) {
alert( "Data Saved: " + msg );
}
});
Solution 2
The dataType
param in jQuery isn't what you send, but rather specifies the format you expect the answer to be (yes, that's a very poor name). If you want to send your data to the server in a format other then application/x-www-form-urlencoded
you should use contentType
param. You also need to serialize your data
:
$.ajax({
type: "PUT",
url: '/admin/pages/1.json',
data: JSON.stringify({...}),
contentType: 'application/json', // format of request payload
dataType: 'json', // format of the response
success: function(msg) {
alert( "Data Saved: " + msg );
}
});
Solution 3
var id = $('#route_id').val()
$.ajax({
type: 'PUT',
url: '/routes/'+ id,
data: $('#markerform').serializeArray(),
dataType: "JSON",
success: function(data) {
console.log(data);
}
});
Solution 4
I had a similar problem [object Object] with jQuery/rails, but with HTML, rather than JSON. Perhaps this will help -
param of [object Object]
data: { lesson: { date: drop_date } }
param of lesson[date]
data: { "lesson[date]": drop_date }
hope this helps -
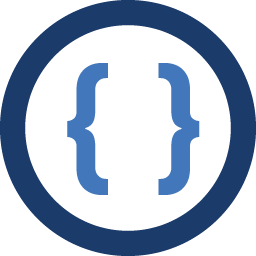
Admin
Updated on October 28, 2020Comments
-
Admin over 3 years
I am trying to send a jquery ajax PUT request that looks like this:
$.ajax({ type: "PUT", url: '/admin/pages/1.json', data: { page : {...} }, dataType: 'json', success: function(msg) { alert( "Data Saved: " + msg ); } });
but I get the following error:
The error occurred while evaluating nil.name /Library/Ruby/Gems/1.8/gems/activesupport-2.3.2/lib/active_support/xml_mini/rexml.rb:29:in `merge_element!' /Library/Ruby/Gems/1.8/gems/activesupport-2.3.2/lib/active_support/xml_mini/rexml.rb:18:in `parse' (__DELEGATION__):2:in `__send__' (__DELEGATION__):2:in `parse' /Library/Ruby/Gems/1.8/gems/activesupport-2.3.2/lib/active_support/core_ext/hash/conversions.rb:154:in `from_xml' ... ...
Is like Rails is trying to parse the params as XML, but I want to use JSON!!
What shall I do to put JSON to rails?
-
Matthew Flaschen almost 15 yearsThat doesn't seem to be the issue here (and do any modern web browsers really lack support for PUT?). The server /is/ receiving the data, just not parsing it the right way.
-
Kris almost 15 yearsyes most browsers lack PUT and DELETE, only GET and POST. The server could be getting the data on the wrong action, ie. if its a POST it would normally map to the Create action and a PUT would map to the Update action.
-
mogsie almost 14 yearsannevankesteren.nl/2007/10/http-method-support would have me believing otherwise (i.e. that a lot of browsers actually do support PUT and DELETE).
-
Marc-André Lafortune almost 12 yearsThe
data
is wrong anddataType
is not what needs to be set. Lost an hour because of this answer. -
Renaud63 almost 12 yearsThis didn't work for me, the comment below gave the correct answer: use JSON.stringify on the sent data, and set contentType (not dataType!) to 'application/json'.
-
Pascal over 11 years@Marc-André Lafortune: so what is the correct answer here or what did not work for you?
-
Marc-André Lafortune over 11 years@pascalbetz: The correct answer is lqc's. This answer will not make Rails parse this request as json, it will only return json.
-
Hengjie about 11 yearsCould you name the browsers lack
PUT/DELETE
? It appears that all of them support it and it's been part of the early HTML 1.1 spec. -
Yarin over 10 years@Kris- you're wrong- all modern browsers support PUT and DELETE via AJAX, just not with HTML forms. See lqc's answer on why this isn't working.