How do I rename a filename after uploading with php?
Solution 1
When using move_uploaded_file
you get to pick the filename, so you can pick anything you want.
When you upload the file, its put into a temporary directory with a temporary name, move_uploaded_file()
allows you to move that file and in that you need to set the name of the file as well.
Solution 2
I'd suggest you to check out Verot's File Upload class. It heals a lot of the pain of file uploading via php, and makes your code much more readable / maintainable.
Here is the link to the class and documentation.
As to the precise answer to your question: To give a new name to an uploaded file, put it as second argument to your move_uploaded_file() function.
Since you want to keep the extension, store it first in a variable:
$ext = explode('.',$_FILES['uploaded']['name']);
$extension = $ext[1];
You could use the file root name to generate the new name (here, with a timestamp appended to it):
$newname = $ext[0].'_'.time();
Say you uploaded a file, and your form input variable name is "myfile.png" it will become 'myfile_2343544.png';
Now, combine the local path to the target directory, the $newname var and the file's extension to set the function's second argument:
$full_local_path = 'path/to/your/filefolder/'.$newname.'.'.$extension ;
move_uploaded_file($_FILES['uploaded']['tmp_name'], $full_local_path);
Solution 3
Why not just save it with a different name:
Just change the name of the $uploadfile variable:
$uploadfile = $uploaddir . "somefilename" . end(explode(".", $file['name']));
It wouldn't hurt however if you refactord your code a little.
Solution 4
When you do
$uploadfile = $uploaddir . basename($_FILES['userfile']['name']);
if (move_uploaded_file($_FILES['userfile']['tmp_name'], $uploadfile)) {
Just set $uploadfile to the whole path of the desired filename; i.e. "uploads/mynewfilename.jpg"
Solution 5
You can use the second param in move_uploaded_file to set the filename. The first param is the filename of the file in your /tmp folder and the second param is the file dir and the new filename.
move_uploaded_file($_FILES['file']['tmp_name'], "/new/file/dir/".[the new file name]);
I know this is an old question but there wasn't a clear answer.
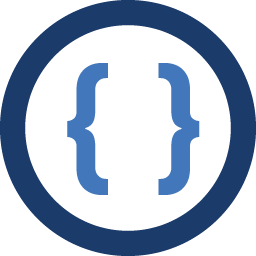
Admin
Updated on July 28, 2022Comments
-
Admin almost 2 years
How do I rename the file either before or after it gets uploaded? I just want to rename the filename, not the extension.
$changeTXT = $_SESSION['username']; $uploaderName = strtolower($changeTXT); $changeTXT = strtolower($changeTXT); $changeTXT = ucfirst($changeTXT); $filelocation = $_POST['userfile']; $filename = $_POST['filename']; $max_size = $_POST['MAX_FILE_SIZE']; $file = $_FILES['userfile']; $allowedExtensions = array("wma", "mp3", "wav"); function isAllowedExtension($fileName) { global $allowedExtensions; return in_array(end(explode(".", $fileName)), $allowedExtensions); } if($file['error'] == UPLOAD_ERR_OK) { if(isAllowedExtension($file['name'])) { $uploaddir = "uploads/".$uploaderName."/"; $uploadfile = $uploaddir . basename($_FILES['userfile']['name']); if (move_uploaded_file($_FILES['userfile']['tmp_name'], $uploadfile)) { echo "Thank you for uploading your music!<br /><br />"; } else { echo "Your file did not upload.<br /><br />"; } echo "\n"; echo "<a href='index.php'>Return</a> to index.<br /><br />$uploaddir"; } else { echo "You have tried to upload an invalid file type.<br /><br />"; } } else die("Cannot upload");
-
Magnilex over 9 yearsIt is always helpful if you also explain the answer. It will make your answer even better, and more likely to get upvoted.
-
Cyprus106 almost 9 yearsAlllllmost. But you need $newname.".$extension", adding a period between newname and extension, otherwise it comes out "filenamejpg". Upvoted!
-
pixeline almost 9 yearsI lost myself in the space between the dots... Nice catch, thanks!!