How do i request mic record permission from user
Solution 1
In the new iOS7 it's very simple try this:
if([[AVAudioSession sharedInstance] respondsToSelector:@selector(requestRecordPermission)])
{
[[AVAudioSession sharedInstance] requestRecordPermission];
}
Solution 2
Here is final code snippet that does work for me. It support both Xcode 4 and 5, and works for iOS5+.
#ifndef __IPHONE_7_0
typedef void (^PermissionBlock)(BOOL granted);
#endif
PermissionBlock permissionBlock = ^(BOOL granted) {
if (granted)
{
[self doActualRecording];
}
else
{
// Warn no access to microphone
}
};
// iOS7+
if([[AVAudioSession sharedInstance] respondsToSelector:@selector(requestRecordPermission:)])
{
[[AVAudioSession sharedInstance] performSelector:@selector(requestRecordPermission:)
withObject:permissionBlock];
}
else
{
[self doActualRecording];
}
Solution 3
As "One Man Crew" claimed you can use requestRecordPermission.
Important thing to be aware of is that you must check that requestRecordPermission is implemented. The reason is that if your app would run on older iOS version (iOS 6.x for example) it would crash after this call. To prevent that you must check that this selector is implemented (this is a good practice anyway).
Code should be something like this:
if([[AVAudioSession sharedInstance] respondsToSelector:@selector(requestRecordPermission:)]){
[[AVAudioSession sharedInstance] requestRecordPermission];
}
Using this method your app would support the new OS and also previous versions of the OS.
I'm using this method every time Apple add more functionality to new OS (that way I can support older versions pretty easy).
Solution 4
AVAudioSession.sharedInstance().requestRecordPermission({ (granted) -> Void in
if !granted
{
let microphoneAccessAlert = UIAlertController(title: NSLocalizedString("recording_mic_access",comment:""), message: NSLocalizedString("recording_mic_access_message",comment:""), preferredStyle: UIAlertControllerStyle.Alert)
var okAction = UIAlertAction(title: NSLocalizedString("OK",comment:""), style: UIAlertActionStyle.Default, handler: { (alert: UIAlertAction!) -> Void in
UIApplication.sharedApplication().openURL(NSURL(string: UIApplicationOpenSettingsURLString)!)
})
var cancelAction = UIAlertAction(title: NSLocalizedString("Cancel",comment:""), style: UIAlertActionStyle.Cancel, handler: { (alert: UIAlertAction!) -> Void in
})
microphoneAccessAlert.addAction(okAction)
microphoneAccessAlert.addAction(cancelAction)
self.presentViewController(microphoneAccessAlert, animated: true, completion: nil)
return
}
self.performSegueWithIdentifier("segueNewRecording", sender: nil)
});
Solution 5
Based on https://stackoverflow.com/users/1071887/idan's response.
AVAudioSession *session = [AVAudioSession sharedInstance];
// AZ DEBUG @@ iOS 7+
AVAudioSessionRecordPermission sessionRecordPermission = [session recordPermission];
switch (sessionRecordPermission) {
case AVAudioSessionRecordPermissionUndetermined:
NSLog(@"Mic permission indeterminate. Call method for indeterminate stuff.");
break;
case AVAudioSessionRecordPermissionDenied:
NSLog(@"Mic permission denied. Call method for denied stuff.");
break;
case AVAudioSessionRecordPermissionGranted:
NSLog(@"Mic permission granted. Call method for granted stuff.");
break;
default:
break;
}
Related videos on Youtube
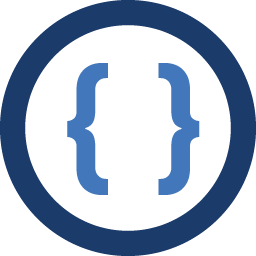
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am using the new iOS7 developer SDK and now the app request from the user his permission to record from mic when the App try to record in the first time.
My App will record after a countdown,so the user can't see this request. I use this code to check the
requestRecordPermission
:[[AVAudioSession sharedInstance] requestRecordPermission:^(BOOL granted) { if (granted) { // Microphone enabled code } else { // Microphone disabled code } }];
But how can i trigger the request by myself before i start to record ?
-
Andrew Barber almost 11 years@OneManCrew The question does not appear to show any effort toward solving the problem themselves, and it basically is asking for a tutorial. (Hence "overly broad" in the close reason)
-
-
One Man Crew almost 11 yearsIt trivial!by the way if you use this method on earlier iOS versions the method will do nothing,because the method is part of the ios7 sdk.
-
Idan almost 11 yearsIt's not reasonable to use this method with previous SDKs because you won't compile to iOS 7 and you have nothing to do with it. But if you are using the new SDK and target older devices (iOS 6.x for example), If you won't use this check your app would definitely crash! So, Please pay attention to use this super important check. It happens every time Apple adds a new feature. If it was the other way (Apple remove feature) your app won't crash and you would just get a warning that the function is deprecated (no crash).
-
Admin almost 11 yearsYou are very wrong! There is no direct connection between the SDK package with which was built the application and the operating system installed on the device.You can build complete apps with the SDK of IOS7 and it will run on older OS.!!
-
Idan almost 11 yearsI think you misunderstood me. To be plain simple, If you use: requestRecordPermission on SDK before 7.x your app won't compile (any Xcode before Xcode 5). If you use SDK 7.x (Xcode 5.x) and don't implement the check on the answer your app would crash on any iOS before 7.x (iOS 5.x or iOS 6.x) Hope that is more clear now.
-
Admin almost 11 yearsI tried it and i don't implement the check on iPhone 4S iOS 6.1 and the app not crash, i think you wrong.
-
C0D3 almost 11 yearsActually in iOS7 this function requires usage with blocks which can be called like this: [[AVAudioSession sharedInstance] requestRecordPermission:^(BOOL response){ NSLog(@"Allow microphone use response: %d", response); }];
-
C0D3 almost 11 yearsAlso you might need a #ifdef __IPHONE_7_0 around the above code #endif to compile it in older versions of xcode.
-
user523234 over 10 yearsThe PrivacyPrompts sample app from Apple utilized the requestRecordPermission is not working. What gives?
-
PK86 over 10 yearscan you please check this question? stackoverflow.com/questions/18957643/… and provide needful information, Thanks
-
Alex Zavatone over 8 yearsThis approach works great on from iOS 7+ on to iOS 9.x. I'll put together a response based on this.